mirror of
https://github.com/pelican-dev/panel.git
synced 2025-05-20 00:34:44 +02:00
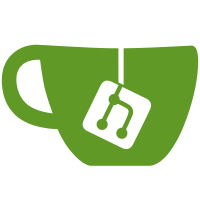
* add spatie/permissions * add policies * add role resource * add root admin role handling * replace some "root_admin" with function * add model specific permissions * make permission selection nicer * fix user creation * fix tests * add back subuser checks in server policy * add custom model for role * assign new users to role if root_admin is set * add api for roles * fix phpstan * add permissions for settings page * remove "restore" and "forceDelete" permissions * add user count to list * prevent deletion if role has users * update user list * fix server policy * remove old `root_admin` column * small refactor * fix tests * forgot can checks here * forgot use * disable editing own roles & disable assigning root admin * don't allow to rename root admin role * remove php bombing exception handler * fix role assignment when creating a user * fix disableOptionWhen * fix missing `root_admin` attribute on react frontend * add permission check for bulk delete * rename viewAny to viewList * improve canAccessPanel check * fix admin not displaying for non-root admins * make sure non root admins can't edit root admins * fix import * fix settings page permission check * fix server permissions for non-subusers * fix settings page permission check v2 * small cleanup * cleanup config file * move consts from resouce into enum & model * Update database/migrations/2024_08_01_114538_remove_root_admin_column.php Co-authored-by: Lance Pioch <lancepioch@gmail.com> * fix config * fix phpstan * fix phpstan 2.0 --------- Co-authored-by: Lance Pioch <lancepioch@gmail.com>
53 lines
1.4 KiB
PHP
53 lines
1.4 KiB
PHP
<?php
|
|
|
|
namespace App\Tests\Unit\Http\Middleware\Api\Application;
|
|
|
|
use App\Tests\Unit\Http\Middleware\MiddlewareTestCase;
|
|
use Symfony\Component\HttpKernel\Exception\AccessDeniedHttpException;
|
|
use App\Http\Middleware\Api\Application\AuthenticateApplicationUser;
|
|
|
|
class AuthenticateUserTest extends MiddlewareTestCase
|
|
{
|
|
/**
|
|
* Test that no user defined results in an access denied exception.
|
|
*/
|
|
public function testNoUserDefined(): void
|
|
{
|
|
$this->expectException(AccessDeniedHttpException::class);
|
|
|
|
$this->setRequestUserModel(null);
|
|
|
|
$this->getMiddleware()->handle($this->request, $this->getClosureAssertions());
|
|
}
|
|
|
|
/**
|
|
* Test that a non-admin user results in an exception.
|
|
*/
|
|
public function testNonAdminUser(): void
|
|
{
|
|
$this->expectException(AccessDeniedHttpException::class);
|
|
|
|
$this->generateRequestUserModel(false);
|
|
|
|
$this->getMiddleware()->handle($this->request, $this->getClosureAssertions());
|
|
}
|
|
|
|
/**
|
|
* Test that an admin user continues though the middleware.
|
|
*/
|
|
public function testAdminUser(): void
|
|
{
|
|
$this->generateRequestUserModel(true);
|
|
|
|
$this->getMiddleware()->handle($this->request, $this->getClosureAssertions());
|
|
}
|
|
|
|
/**
|
|
* Return an instance of the middleware for testing.
|
|
*/
|
|
private function getMiddleware(): AuthenticateApplicationUser
|
|
{
|
|
return new AuthenticateApplicationUser();
|
|
}
|
|
}
|