mirror of
https://github.com/pelican-dev/panel.git
synced 2025-07-02 20:21:08 +02:00
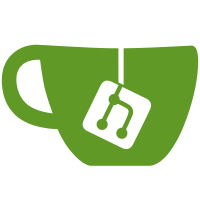
* Init * Health Page * Admin API Keys * Update API Keys * Database Hosts * Mounts * remove `s` * Users * Webhooks * Server never again... * Fix Server * Settings * Update Mounts * Update Databasehost * Update Server * Oops, Update Server * Nodes * Update User * Dashboard * Update Server * Profile * Egg * Role & Update Egg * Add base Laravel lang files * update apikey * remove html back to settings, remove comment * add `:resource` to create_action * Update Egg * Update Egg v2 * Update 1 * trans cf info label * Update charts * more trans * Update Webhook * update Health * Update Server * Update Role * Fixes * Bulk Update * AnotherOne * Fix relation button label * rename `admin1` to `admin` Leftover from testing... oops * More Translations * Updates * `pint` + Relation Manager Titles
57 lines
1.7 KiB
PHP
57 lines
1.7 KiB
PHP
<?php
|
|
|
|
namespace App\Filament\Admin\Resources\RoleResource\Pages;
|
|
|
|
use App\Filament\Admin\Resources\RoleResource;
|
|
use App\Models\Role;
|
|
use Filament\Actions\DeleteAction;
|
|
use Filament\Resources\Pages\EditRecord;
|
|
use Illuminate\Support\Arr;
|
|
use Illuminate\Support\Collection;
|
|
use Spatie\Permission\Models\Permission;
|
|
|
|
/**
|
|
* @property Role $record
|
|
*/
|
|
class EditRole extends EditRecord
|
|
{
|
|
protected static string $resource = RoleResource::class;
|
|
|
|
public Collection $permissions;
|
|
|
|
protected function mutateFormDataBeforeSave(array $data): array
|
|
{
|
|
$this->permissions = collect($data)
|
|
->filter(function ($permission, $key) {
|
|
return !in_array($key, ['name', 'guard_name']);
|
|
})
|
|
->values()
|
|
->flatten()
|
|
->unique();
|
|
|
|
return Arr::only($data, ['name', 'guard_name']);
|
|
}
|
|
|
|
protected function afterSave(): void
|
|
{
|
|
$permissionModels = collect();
|
|
$this->permissions->each(function ($permission) use ($permissionModels) {
|
|
$permissionModels->push(Permission::firstOrCreate([
|
|
'name' => $permission,
|
|
'guard_name' => $this->data['guard_name'],
|
|
]));
|
|
});
|
|
|
|
$this->record->syncPermissions($permissionModels);
|
|
}
|
|
|
|
protected function getHeaderActions(): array
|
|
{
|
|
return [
|
|
DeleteAction::make()
|
|
->disabled(fn (Role $role) => $role->isRootAdmin() || $role->users_count >= 1)
|
|
->label(fn (Role $role) => $role->isRootAdmin() ? trans('admin/role.root_admin_delete') : ($role->users_count >= 1 ? trans('admin/role.in_use') : trans('filament-actions::delete.single.label'))),
|
|
];
|
|
}
|
|
}
|