mirror of
https://github.com/pelican-dev/panel.git
synced 2025-07-12 12:01:09 +02:00
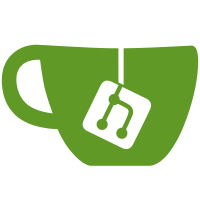
* Init * Health Page * Admin API Keys * Update API Keys * Database Hosts * Mounts * remove `s` * Users * Webhooks * Server never again... * Fix Server * Settings * Update Mounts * Update Databasehost * Update Server * Oops, Update Server * Nodes * Update User * Dashboard * Update Server * Profile * Egg * Role & Update Egg * Add base Laravel lang files * update apikey * remove html back to settings, remove comment * add `:resource` to create_action * Update Egg * Update Egg v2 * Update 1 * trans cf info label * Update charts * more trans * Update Webhook * update Health * Update Server * Update Role * Fixes * Bulk Update * AnotherOne * Fix relation button label * rename `admin1` to `admin` Leftover from testing... oops * More Translations * Updates * `pint` + Relation Manager Titles
79 lines
1.9 KiB
PHP
79 lines
1.9 KiB
PHP
<?php
|
|
|
|
namespace App\Checks;
|
|
|
|
use Carbon\Carbon;
|
|
use Composer\InstalledVersions;
|
|
use Spatie\Health\Checks\Check;
|
|
use Spatie\Health\Checks\Result;
|
|
|
|
class ScheduleCheck extends Check
|
|
{
|
|
protected string $cacheKey = 'health:checks:schedule:latestHeartbeatAt';
|
|
|
|
protected ?string $cacheStoreName = null;
|
|
|
|
protected int $heartbeatMaxAgeInMinutes = 1;
|
|
|
|
public function useCacheStore(string $cacheStoreName): self
|
|
{
|
|
$this->cacheStoreName = $cacheStoreName;
|
|
|
|
return $this;
|
|
}
|
|
|
|
public function getCacheStoreName(): string
|
|
{
|
|
return $this->cacheStoreName ?? config('cache.default');
|
|
}
|
|
|
|
public function cacheKey(string $cacheKey): self
|
|
{
|
|
$this->cacheKey = $cacheKey;
|
|
|
|
return $this;
|
|
}
|
|
|
|
public function heartbeatMaxAgeInMinutes(int $heartbeatMaxAgeInMinutes): self
|
|
{
|
|
$this->heartbeatMaxAgeInMinutes = $heartbeatMaxAgeInMinutes;
|
|
|
|
return $this;
|
|
}
|
|
|
|
public function getCacheKey(): string
|
|
{
|
|
return $this->cacheKey;
|
|
}
|
|
|
|
public function run(): Result
|
|
{
|
|
$result = Result::make()->ok(trans('admin/health.results.schedule.ok'));
|
|
|
|
$lastHeartbeatTimestamp = cache()->store($this->cacheStoreName)->get($this->cacheKey);
|
|
|
|
if (!$lastHeartbeatTimestamp) {
|
|
return $result->failed(trans('admin/health.results.schedule.failed_not_ran'));
|
|
}
|
|
|
|
$latestHeartbeatAt = Carbon::createFromTimestamp($lastHeartbeatTimestamp);
|
|
|
|
$carbonVersion = InstalledVersions::getVersion('nesbot/carbon');
|
|
|
|
$minutesAgo = $latestHeartbeatAt->diffInMinutes();
|
|
|
|
if (version_compare($carbonVersion,
|
|
'3.0.0', '<')) {
|
|
$minutesAgo += 1;
|
|
}
|
|
|
|
if ($minutesAgo > $this->heartbeatMaxAgeInMinutes) {
|
|
return $result->failed(trans('admin/health.results.schedule.failed_last_ran', [
|
|
'time' => $minutesAgo,
|
|
]));
|
|
}
|
|
|
|
return $result;
|
|
}
|
|
}
|