mirror of
https://github.com/pelican-dev/panel.git
synced 2025-05-19 22:14:45 +02:00
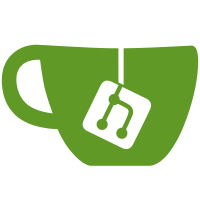
* add back network chart * don't show timestamp * convert "total" to "real time" * fix typo * set min to 0 * sort data to make sure we actually get the previous value * Fix `ServerNetworkChart` * Many changes... * small cleanup --------- Co-authored-by: RMartinOscar <40749467+RMartinOscar@users.noreply.github.com> Co-authored-by: notCharles <charles@pelican.dev>
77 lines
1.9 KiB
PHP
77 lines
1.9 KiB
PHP
<?php
|
|
|
|
namespace App\Filament\Server\Widgets;
|
|
|
|
use App\Models\Server;
|
|
use Carbon\Carbon;
|
|
use Filament\Support\RawJs;
|
|
use Filament\Widgets\ChartWidget;
|
|
use Illuminate\Support\Number;
|
|
|
|
class ServerCpuChart extends ChartWidget
|
|
{
|
|
protected static ?string $pollingInterval = '1s';
|
|
|
|
protected static ?string $maxHeight = '200px';
|
|
|
|
public ?Server $server = null;
|
|
|
|
protected function getData(): array
|
|
{
|
|
$period = auth()->user()->getCustomization()['console_graph_period'] ?? 30;
|
|
$cpu = collect(cache()->get("servers.{$this->server->id}.cpu_absolute"))
|
|
->slice(-$period)
|
|
->map(fn ($value, $key) => [
|
|
'cpu' => Number::format($value, maxPrecision: 2),
|
|
'timestamp' => Carbon::createFromTimestamp($key, auth()->user()->timezone ?? 'UTC')->format('H:i:s'),
|
|
])
|
|
->all();
|
|
|
|
return [
|
|
'datasets' => [
|
|
[
|
|
'data' => array_column($cpu, 'cpu'),
|
|
'backgroundColor' => [
|
|
'rgba(96, 165, 250, 0.3)',
|
|
],
|
|
'tension' => '0.3',
|
|
'fill' => true,
|
|
],
|
|
],
|
|
'labels' => array_column($cpu, 'timestamp'),
|
|
'locale' => auth()->user()->language ?? 'en',
|
|
];
|
|
}
|
|
|
|
protected function getType(): string
|
|
{
|
|
return 'line';
|
|
}
|
|
|
|
protected function getOptions(): RawJs
|
|
{
|
|
return RawJs::make(<<<'JS'
|
|
{
|
|
scales: {
|
|
y: {
|
|
min: 0,
|
|
},
|
|
x: {
|
|
display: false,
|
|
}
|
|
},
|
|
plugins: {
|
|
legend: {
|
|
display: false,
|
|
}
|
|
}
|
|
}
|
|
JS);
|
|
}
|
|
|
|
public function getHeading(): string
|
|
{
|
|
return 'CPU';
|
|
}
|
|
}
|