mirror of
https://github.com/pelican-dev/panel.git
synced 2025-07-10 09:51:08 +02:00
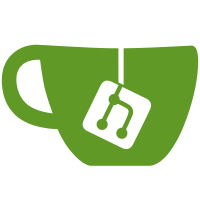
* allow importing eggs via url * refactor * run pint * turn back into one button * fix empty check * small cleanup * removed container for tabs * Update URL function * Use sys temp --------- Co-authored-by: notCharles <charles@pelican.dev>
68 lines
2.2 KiB
PHP
68 lines
2.2 KiB
PHP
<?php
|
|
|
|
namespace App\Services\Eggs\Sharing;
|
|
|
|
use App\Models\Egg;
|
|
use Illuminate\Http\UploadedFile;
|
|
use Illuminate\Support\Collection;
|
|
use App\Models\EggVariable;
|
|
use Illuminate\Database\ConnectionInterface;
|
|
use App\Services\Eggs\EggParserService;
|
|
use Spatie\TemporaryDirectory\TemporaryDirectory;
|
|
|
|
class EggUpdateImporterService
|
|
{
|
|
/**
|
|
* EggUpdateImporterService constructor.
|
|
*/
|
|
public function __construct(protected ConnectionInterface $connection, protected EggParserService $parser)
|
|
{
|
|
}
|
|
|
|
/**
|
|
* Update an existing Egg using an uploaded JSON file.
|
|
*
|
|
* @throws \App\Exceptions\Service\InvalidFileUploadException|\Throwable
|
|
*/
|
|
public function fromFile(Egg $egg, UploadedFile $file): Egg
|
|
{
|
|
$parsed = $this->parser->handle($file);
|
|
|
|
return $this->connection->transaction(function () use ($egg, $parsed) {
|
|
$egg = $this->parser->fillFromParsed($egg, $parsed);
|
|
$egg->save();
|
|
|
|
// Update existing variables or create new ones.
|
|
foreach ($parsed['variables'] ?? [] as $variable) {
|
|
EggVariable::unguarded(function () use ($egg, $variable) {
|
|
$egg->variables()->updateOrCreate([
|
|
'env_variable' => $variable['env_variable'],
|
|
], Collection::make($variable)->except(['egg_id', 'env_variable'])->toArray());
|
|
});
|
|
}
|
|
|
|
$imported = array_map(fn ($value) => $value['env_variable'], $parsed['variables'] ?? []);
|
|
|
|
$egg->variables()->whereNotIn('env_variable', $imported)->delete();
|
|
|
|
return $egg->refresh();
|
|
});
|
|
}
|
|
|
|
/**
|
|
* Update an existing Egg using an url.
|
|
*
|
|
* @throws \App\Exceptions\Service\InvalidFileUploadException|\Throwable
|
|
*/
|
|
public function fromUrl(Egg $egg, string $url): Egg
|
|
{
|
|
$info = pathinfo($url);
|
|
$tmpDir = TemporaryDirectory::make()->deleteWhenDestroyed();
|
|
$tmpPath = $tmpDir->path($info['basename']);
|
|
|
|
file_put_contents($tmpPath, file_get_contents($url));
|
|
|
|
return $this->fromFile($egg, new UploadedFile($tmpPath, $info['basename'], 'application/json'));
|
|
}
|
|
}
|