mirror of
https://github.com/pelican-dev/panel.git
synced 2025-05-19 23:24:46 +02:00
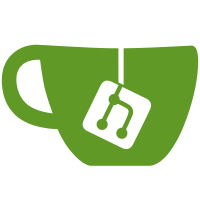
* use RESOURCE_NAME for requests * use RESOURCE_NAME for transformers * add permissions field to api key * add migration for new permissions field * update tests * remove debug log * set column type to "json" * remove default attribute to fix tests * fix default value for permissions * fix after merge * fix after merge * allow to "register" custom permissions * add "role" to default resource names * fix after merge * fix phpstan * fix migrations
48 lines
1.1 KiB
PHP
48 lines
1.1 KiB
PHP
<?php
|
|
|
|
namespace App\Services\Acl\Api;
|
|
|
|
use App\Models\ApiKey;
|
|
|
|
class AdminAcl
|
|
{
|
|
/**
|
|
* The different types of permissions available for API keys. This
|
|
* implements a read/write/none permissions scheme for all endpoints.
|
|
*/
|
|
public const NONE = 0;
|
|
|
|
public const READ = 1;
|
|
|
|
public const WRITE = 2;
|
|
|
|
/**
|
|
* Determine if an API key has permission to perform a specific read/write operation.
|
|
*/
|
|
public static function can(int $permission, int $action = self::READ): bool
|
|
{
|
|
if ($permission & $action) {
|
|
return true;
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
/**
|
|
* Determine if an API Key model has permission to access a given resource
|
|
* at a specific action level.
|
|
*/
|
|
public static function check(ApiKey $key, string $resource, int $action = self::READ): bool
|
|
{
|
|
return self::can($key->getPermission($resource), $action);
|
|
}
|
|
|
|
/**
|
|
* Returns a list of all possible permissions.
|
|
*/
|
|
public static function getResourceList(): array
|
|
{
|
|
return ApiKey::getPermissionList();
|
|
}
|
|
}
|