mirror of
https://github.com/pelican-dev/panel.git
synced 2025-07-04 00:21:07 +02:00
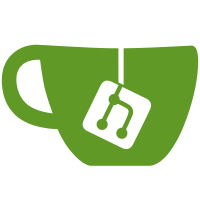
* Fix these * Update phpstan * Transform these into their identifiers instead * Fix custom rule * License is wrong * Update these * Pint fixes * Fix this * Consolidate these * Never supported PHP 7 * Better evaluation * Fixes * Don’t need ignore * Replace trait with service * Subusers are simply the many to many relationship between Servers and Users * Adjust to remove ignores * Use new query builder instead! * wip * Update composer * Quick fixes * Use realtime facade * Small fixes * Convert to static to avoid new * Update to statics * Don’t modify protected properties directly * Run pint * Change to correct method * Give up and use the facade * Make sure this route is available * Filament hasn’t been loaded yet * This can be readonly * Typehint * These are no longer used * Quick fixes * Need doc block help * Always true * We use caddy with docker * Pint * Fix phpstan issues * Remove unused import --------- Co-authored-by: MartinOscar <40749467+RMartinOscar@users.noreply.github.com>
43 lines
1.1 KiB
PHP
43 lines
1.1 KiB
PHP
<?php
|
|
|
|
namespace App\PHPStan;
|
|
|
|
use PhpParser\Node;
|
|
use PhpParser\Node\Expr\FuncCall;
|
|
use PHPStan\Analyser\Scope;
|
|
use PHPStan\Rules\Rule;
|
|
use PHPStan\Rules\RuleErrorBuilder;
|
|
|
|
class ForbiddenGlobalFunctionsRule implements Rule
|
|
{
|
|
private array $forbiddenFunctions;
|
|
|
|
public function __construct(array $forbiddenFunctions = ['app', 'resolve'])
|
|
{
|
|
$this->forbiddenFunctions = $forbiddenFunctions;
|
|
}
|
|
|
|
public function getNodeType(): string
|
|
{
|
|
return FuncCall::class;
|
|
}
|
|
|
|
public function processNode(Node $node, Scope $scope): array
|
|
{
|
|
/** @var FuncCall $node */
|
|
if ($node->name instanceof Node\Name) {
|
|
$functionName = (string) $node->name;
|
|
if (in_array($functionName, $this->forbiddenFunctions, true)) {
|
|
return [
|
|
RuleErrorBuilder::message(sprintf(
|
|
'Usage of global function "%s" is forbidden.',
|
|
$functionName,
|
|
))->identifier('myCustomRules.forbiddenGlobalFunctions')->build(),
|
|
];
|
|
}
|
|
}
|
|
|
|
return [];
|
|
}
|
|
}
|