mirror of
https://github.com/pelican-dev/panel.git
synced 2025-05-19 21:04:44 +02:00
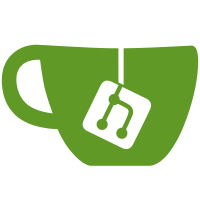
* Fix these * Update phpstan * Transform these into their identifiers instead * Fix custom rule * License is wrong * Update these * Pint fixes * Fix this * Consolidate these * Never supported PHP 7 * Better evaluation * Fixes * Don’t need ignore * Replace trait with service * Subusers are simply the many to many relationship between Servers and Users * Adjust to remove ignores * Use new query builder instead! * wip * Update composer * Quick fixes * Use realtime facade * Small fixes * Convert to static to avoid new * Update to statics * Don’t modify protected properties directly * Run pint * Change to correct method * Give up and use the facade * Make sure this route is available * Filament hasn’t been loaded yet * This can be readonly * Typehint * These are no longer used * Quick fixes * Need doc block help * Always true * We use caddy with docker * Pint * Fix phpstan issues * Remove unused import --------- Co-authored-by: MartinOscar <40749467+RMartinOscar@users.noreply.github.com>
77 lines
2.2 KiB
PHP
77 lines
2.2 KiB
PHP
<?php
|
|
|
|
namespace App\Http\Controllers\Auth;
|
|
|
|
use App\Extensions\OAuth\Providers\OAuthProvider;
|
|
use App\Filament\Pages\Auth\EditProfile;
|
|
use Filament\Notifications\Notification;
|
|
use Illuminate\Auth\AuthManager;
|
|
use Illuminate\Http\RedirectResponse;
|
|
use Laravel\Socialite\Facades\Socialite;
|
|
use App\Http\Controllers\Controller;
|
|
use App\Models\User;
|
|
use App\Services\Users\UserUpdateService;
|
|
use Exception;
|
|
use Illuminate\Http\Request;
|
|
|
|
class OAuthController extends Controller
|
|
{
|
|
public function __construct(
|
|
private readonly AuthManager $auth,
|
|
private readonly UserUpdateService $updateService
|
|
) {}
|
|
|
|
/**
|
|
* Redirect user to the OAuth provider
|
|
*/
|
|
protected function redirect(string $driver): RedirectResponse
|
|
{
|
|
// Driver is disabled - redirect to normal login
|
|
if (!OAuthProvider::get($driver)->isEnabled()) {
|
|
return redirect()->route('auth.login');
|
|
}
|
|
|
|
return Socialite::with($driver)->redirect();
|
|
}
|
|
|
|
/**
|
|
* Callback from OAuth provider.
|
|
*/
|
|
protected function callback(Request $request, string $driver): RedirectResponse
|
|
{
|
|
// Driver is disabled - redirect to normal login
|
|
if (!OAuthProvider::get($driver)->isEnabled()) {
|
|
return redirect()->route('auth.login');
|
|
}
|
|
|
|
$oauthUser = Socialite::driver($driver)->user();
|
|
|
|
// User is already logged in and wants to link a new OAuth Provider
|
|
if ($request->user()) {
|
|
$oauth = $request->user()->oauth;
|
|
$oauth[$driver] = $oauthUser->getId();
|
|
|
|
$this->updateService->handle($request->user(), ['oauth' => $oauth]);
|
|
|
|
return redirect(EditProfile::getUrl(['tab' => '-oauth-tab']));
|
|
}
|
|
|
|
try {
|
|
$user = User::query()->whereJsonContains('oauth->'. $driver, $oauthUser->getId())->firstOrFail();
|
|
|
|
$this->auth->guard()->login($user, true);
|
|
} catch (Exception) {
|
|
// No user found - redirect to normal login
|
|
Notification::make()
|
|
->title('No linked User found')
|
|
->danger()
|
|
->persistent()
|
|
->send();
|
|
|
|
return redirect()->route('auth.login');
|
|
}
|
|
|
|
return redirect('/');
|
|
}
|
|
}
|