mirror of
https://github.com/pelican-dev/panel.git
synced 2025-07-02 07:41:08 +02:00
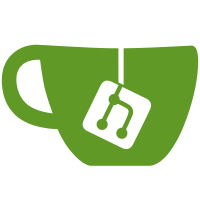
* Fix these * Update phpstan * Transform these into their identifiers instead * Fix custom rule * License is wrong * Update these * Pint fixes * Fix this * Consolidate these * Never supported PHP 7 * Better evaluation * Fixes * Don’t need ignore * Replace trait with service * Subusers are simply the many to many relationship between Servers and Users * Adjust to remove ignores * Use new query builder instead! * wip * Update composer * Quick fixes * Use realtime facade * Small fixes * Convert to static to avoid new * Update to statics * Don’t modify protected properties directly * Run pint * Change to correct method * Give up and use the facade * Make sure this route is available * Filament hasn’t been loaded yet * This can be readonly * Typehint * These are no longer used * Quick fixes * Need doc block help * Always true * We use caddy with docker * Pint * Fix phpstan issues * Remove unused import --------- Co-authored-by: MartinOscar <40749467+RMartinOscar@users.noreply.github.com>
86 lines
2.6 KiB
PHP
86 lines
2.6 KiB
PHP
<?php
|
|
|
|
namespace App\Filament\Components\Tables\Actions;
|
|
|
|
use App\Services\Eggs\Sharing\EggImporterService;
|
|
use Exception;
|
|
use Filament\Forms\Components\FileUpload;
|
|
use Filament\Forms\Components\Tabs;
|
|
use Filament\Forms\Components\Tabs\Tab;
|
|
use Filament\Forms\Components\TextInput;
|
|
use Filament\Notifications\Notification;
|
|
use Filament\Tables\Actions\Action;
|
|
|
|
class ImportEggAction extends Action
|
|
{
|
|
public static function getDefaultName(): ?string
|
|
{
|
|
return 'import';
|
|
}
|
|
|
|
protected function setUp(): void
|
|
{
|
|
parent::setUp();
|
|
|
|
$this->label('Import');
|
|
|
|
$this->authorize(fn () => auth()->user()->can('import egg'));
|
|
|
|
$this->form([
|
|
Tabs::make('Tabs')
|
|
->contained(false)
|
|
->tabs([
|
|
Tab::make('From File')
|
|
->icon('tabler-file-upload')
|
|
->schema([
|
|
FileUpload::make('egg')
|
|
->label('Egg')
|
|
->hint('This should be the json file ( egg-minecraft.json )')
|
|
->acceptedFileTypes(['application/json'])
|
|
->storeFiles(false)
|
|
->multiple(),
|
|
]),
|
|
Tab::make('From URL')
|
|
->icon('tabler-world-upload')
|
|
->schema([
|
|
TextInput::make('url')
|
|
->label('URL')
|
|
->hint('This URL should point to a single json file')
|
|
->url(),
|
|
]),
|
|
]),
|
|
]);
|
|
|
|
$this->action(function (array $data, EggImporterService $eggImportService): void {
|
|
try {
|
|
if (!empty($data['egg'])) {
|
|
$eggFile = $data['egg'];
|
|
|
|
foreach ($eggFile as $file) {
|
|
$eggImportService->fromFile($file);
|
|
}
|
|
}
|
|
|
|
if (!empty($data['url'])) {
|
|
$eggImportService->fromUrl($data['url']);
|
|
}
|
|
} catch (Exception $exception) {
|
|
Notification::make()
|
|
->title('Import Failed')
|
|
->body($exception->getMessage())
|
|
->danger()
|
|
->send();
|
|
|
|
report($exception);
|
|
|
|
return;
|
|
}
|
|
|
|
Notification::make()
|
|
->title('Import Success')
|
|
->success()
|
|
->send();
|
|
});
|
|
}
|
|
}
|