mirror of
https://github.com/pelican-dev/panel.git
synced 2025-07-04 12:31:07 +02:00
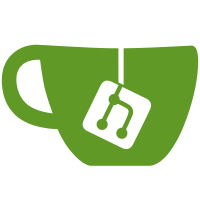
* Fix these * Update phpstan * Transform these into their identifiers instead * Fix custom rule * License is wrong * Update these * Pint fixes * Fix this * Consolidate these * Never supported PHP 7 * Better evaluation * Fixes * Don’t need ignore * Replace trait with service * Subusers are simply the many to many relationship between Servers and Users * Adjust to remove ignores * Use new query builder instead! * wip * Update composer * Quick fixes * Use realtime facade * Small fixes * Convert to static to avoid new * Update to statics * Don’t modify protected properties directly * Run pint * Change to correct method * Give up and use the facade * Make sure this route is available * Filament hasn’t been loaded yet * This can be readonly * Typehint * These are no longer used * Quick fixes * Need doc block help * Always true * We use caddy with docker * Pint * Fix phpstan issues * Remove unused import --------- Co-authored-by: MartinOscar <40749467+RMartinOscar@users.noreply.github.com>
74 lines
2.7 KiB
PHP
74 lines
2.7 KiB
PHP
<?php
|
|
|
|
namespace App\Filament\Admin\Resources\UserResource\Pages;
|
|
|
|
use App\Filament\Admin\Resources\UserResource;
|
|
use App\Models\Role;
|
|
use App\Models\User;
|
|
use App\Services\Helpers\LanguageService;
|
|
use Filament\Actions\DeleteAction;
|
|
use Filament\Forms\Components\CheckboxList;
|
|
use Filament\Forms\Components\Hidden;
|
|
use Filament\Forms\Components\Section;
|
|
use Filament\Forms\Components\Select;
|
|
use Filament\Forms\Components\TextInput;
|
|
use Filament\Forms\Form;
|
|
use Filament\Resources\Pages\EditRecord;
|
|
use Illuminate\Support\Facades\Hash;
|
|
|
|
class EditUser extends EditRecord
|
|
{
|
|
protected static string $resource = UserResource::class;
|
|
|
|
public function form(Form $form): Form
|
|
{
|
|
return $form
|
|
->schema([
|
|
Section::make()->schema([
|
|
TextInput::make('username')
|
|
->required()
|
|
->minLength(3)
|
|
->maxLength(255),
|
|
TextInput::make('email')
|
|
->email()
|
|
->required()
|
|
->maxLength(255),
|
|
TextInput::make('password')
|
|
->dehydrateStateUsing(fn (string $state): string => Hash::make($state))
|
|
->dehydrated(fn (?string $state): bool => filled($state))
|
|
->password(),
|
|
Select::make('language')
|
|
->required()
|
|
->hidden()
|
|
->default('en')
|
|
->options(fn (LanguageService $languageService) => $languageService->getAvailableLanguages()),
|
|
Hidden::make('skipValidation')
|
|
->default(true),
|
|
CheckboxList::make('roles')
|
|
->disabled(fn (User $user) => $user->id === auth()->user()->id)
|
|
->disableOptionWhen(fn (string $value): bool => $value == Role::getRootAdmin()->id)
|
|
->relationship('roles', 'name')
|
|
->label('Admin Roles')
|
|
->columnSpanFull()
|
|
->bulkToggleable(false),
|
|
])
|
|
->columns(['default' => 1, 'lg' => 3]),
|
|
]);
|
|
}
|
|
|
|
protected function getHeaderActions(): array
|
|
{
|
|
return [
|
|
DeleteAction::make()
|
|
->label(fn (User $user) => auth()->user()->id === $user->id ? 'Can\'t Delete Yourself' : ($user->servers()->count() > 0 ? 'User Has Servers' : 'Delete'))
|
|
->disabled(fn (User $user) => auth()->user()->id === $user->id || $user->servers()->count() > 0),
|
|
$this->getSaveFormAction()->formId('form'),
|
|
];
|
|
}
|
|
|
|
protected function getFormActions(): array
|
|
{
|
|
return [];
|
|
}
|
|
}
|