mirror of
https://github.com/pelican-dev/panel.git
synced 2025-06-30 23:51:07 +02:00
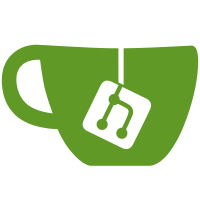
* Not found property rule * Make these “better” * Day 1 * Day 2 * Day 3 * Dat 4 * Remove disabled check * Day 4 continued * Run pint * Final changes hopefully * Pint fixes * Fix again * Reset these * Update app/Filament/Admin/Pages/Health.php Co-authored-by: MartinOscar <40749467+rmartinoscar@users.noreply.github.com> * Update app/Traits/CheckMigrationsTrait.php Co-authored-by: MartinOscar <40749467+rmartinoscar@users.noreply.github.com> --------- Co-authored-by: MartinOscar <40749467+rmartinoscar@users.noreply.github.com>
58 lines
1.9 KiB
PHP
58 lines
1.9 KiB
PHP
<?php
|
|
|
|
namespace App\Transformers\Api\Client;
|
|
|
|
use App\Models\User;
|
|
use App\Models\ActivityLog;
|
|
use Illuminate\Database\Eloquent\Model;
|
|
use League\Fractal\Resource\ResourceAbstract;
|
|
|
|
class ActivityLogTransformer extends BaseClientTransformer
|
|
{
|
|
protected array $availableIncludes = ['actor'];
|
|
|
|
public function getResourceName(): string
|
|
{
|
|
return ActivityLog::RESOURCE_NAME;
|
|
}
|
|
|
|
/**
|
|
* @param ActivityLog $model
|
|
*/
|
|
public function transform($model): array
|
|
{
|
|
return [
|
|
// This is not for security, it is only to provide a unique identifier to
|
|
// the front-end for each entry to improve rendering performance since there
|
|
// is nothing else sufficiently unique to key off at this point.
|
|
'id' => sha1((string) $model->id),
|
|
'batch' => $model->batch,
|
|
'event' => $model->event,
|
|
'is_api' => !is_null($model->api_key_id),
|
|
'ip' => $this->canViewIP($model->actor) ? $model->ip : null,
|
|
'description' => $model->description,
|
|
'properties' => $model->wrapProperties(),
|
|
'has_additional_metadata' => $model->hasAdditionalMetadata(),
|
|
'timestamp' => $model->timestamp->toAtomString(),
|
|
];
|
|
}
|
|
|
|
public function includeActor(ActivityLog $model): ResourceAbstract
|
|
{
|
|
if (!$model->actor instanceof User) {
|
|
return $this->null();
|
|
}
|
|
|
|
return $this->item($model->actor, $this->makeTransformer(UserTransformer::class), User::RESOURCE_NAME);
|
|
}
|
|
|
|
/**
|
|
* Determines if the user can view the IP address in the output either because they are the
|
|
* actor that performed the action, or because they are an administrator on the Panel.
|
|
*/
|
|
protected function canViewIP(?Model $actor = null): bool
|
|
{
|
|
return $actor?->is($this->request->user()) || $this->request->user()->can('seeIps activityLog');
|
|
}
|
|
}
|