mirror of
https://github.com/pelican-dev/panel.git
synced 2025-07-19 02:35:17 +02:00
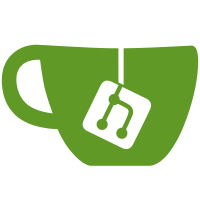
* add application api endpoint to start server transfer * add basics for "cancel transfer" endpoint * wire up wings cancel * lint
45 lines
1.3 KiB
PHP
45 lines
1.3 KiB
PHP
<?php
|
|
|
|
namespace App\Http\Controllers\Admin\Servers;
|
|
|
|
use App\Http\Controllers\Controller;
|
|
use App\Models\Server;
|
|
use App\Services\Servers\TransferServerService;
|
|
use Illuminate\Http\RedirectResponse;
|
|
use Illuminate\Http\Request;
|
|
use Prologue\Alerts\AlertsMessageBag;
|
|
|
|
class ServerTransferController extends Controller
|
|
{
|
|
/**
|
|
* ServerTransferController constructor.
|
|
*/
|
|
public function __construct(
|
|
private AlertsMessageBag $alert,
|
|
private TransferServerService $transferServerService,
|
|
) {
|
|
}
|
|
|
|
/**
|
|
* Starts a transfer of a server to a new node.
|
|
*
|
|
* @throws \Throwable
|
|
*/
|
|
public function transfer(Request $request, Server $server): RedirectResponse
|
|
{
|
|
$validatedData = $request->validate([
|
|
'node_id' => 'required|exists:nodes,id',
|
|
'allocation_id' => 'required|bail|unique:servers|exists:allocations,id',
|
|
'allocation_additional' => 'nullable',
|
|
]);
|
|
|
|
if ($this->transferServerService->handle($server, $validatedData)) {
|
|
$this->alert->success(trans('admin/server.alerts.transfer_started'))->flash();
|
|
} else {
|
|
$this->alert->danger(trans('admin/server.alerts.transfer_not_viable'))->flash();
|
|
}
|
|
|
|
return redirect()->route('admin.servers.view.manage', $server->id);
|
|
}
|
|
}
|