mirror of
https://github.com/pelican-dev/panel.git
synced 2025-05-20 05:14:46 +02:00
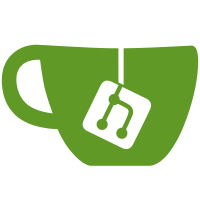
* feat: First Webhook PoC draft * feat: Dispatch Webhooks PoC * fix: typo in webhook configuration scope * Update 2024_04_21_162552_create_webhooks_table.php Co-authored-by: Lance Pioch <lancepioch@gmail.com> * Update 2024_04_21_162552_create_webhooks_table.php Co-authored-by: Lance Pioch <lancepioch@gmail.com> * Update 2024_04_21_162544_create_webhook_configurations_table.php Co-authored-by: Lance Pioch <lancepioch@gmail.com> * Update 2024_04_21_162544_create_webhook_configurations_table.php Co-authored-by: Lance Pioch <lancepioch@gmail.com> * Update DispatchWebhooks.php Co-authored-by: Lance Pioch <lancepioch@gmail.com> * Update DispatchWebhooksJob.php Co-authored-by: Lance Pioch <lancepioch@gmail.com> * Update DispatchWebhookForConfiguration.php Co-authored-by: Lance Pioch <lancepioch@gmail.com> * Update DispatchWebhookForConfiguration.php Co-authored-by: Lance Pioch <lancepioch@gmail.com> * Update DispatchWebhookForConfiguration.php Co-authored-by: Lance Pioch <lancepioch@gmail.com> * Update DispatchWebhooksJob.php Co-authored-by: Lance Pioch <lancepioch@gmail.com> * Update DispatchWebhooksJob.php Co-authored-by: Lance Pioch <lancepioch@gmail.com> * Update DispatchWebhooksJob.php Co-authored-by: Lance Pioch <lancepioch@gmail.com> * chore: Implement Webhook Event Discovery * we got a test working for webhooks * WIP * Something is working! * More tests * clean up the tests now that they are passing * WIP * Don't use model specific events * WIP * WIP * WIP * WIP * WIP * Do it sync * Reset these * Don't need restored event type * Deleted some unused jobs * Find custom Events * Remove observers * Add custom event test * Run Pint * Add caching * Don't cache every single event * Fix tests * Run Pint * Phpstan fixes * Pint fix * Test fixes * Middleware unit test fix * Pint fixes * Remove index not working for older dbs * Use facade instead --------- Co-authored-by: Pascale Beier <mail@pascalebeier.de> Co-authored-by: Lance Pioch <lancepioch@gmail.com> Co-authored-by: Vehikl <go@vehikl.com>
79 lines
2.8 KiB
PHP
79 lines
2.8 KiB
PHP
<?php
|
|
|
|
namespace App\Services\Subusers;
|
|
|
|
use App\Models\User;
|
|
use App\Notifications\AddedToServer;
|
|
use Illuminate\Support\Str;
|
|
use App\Models\Server;
|
|
use App\Models\Subuser;
|
|
use Illuminate\Database\ConnectionInterface;
|
|
use App\Services\Users\UserCreationService;
|
|
use App\Exceptions\Service\Subuser\UserIsServerOwnerException;
|
|
use App\Exceptions\Service\Subuser\ServerSubuserExistsException;
|
|
|
|
class SubuserCreationService
|
|
{
|
|
/**
|
|
* SubuserCreationService constructor.
|
|
*/
|
|
public function __construct(
|
|
private ConnectionInterface $connection,
|
|
private UserCreationService $userCreationService,
|
|
) {
|
|
}
|
|
|
|
/**
|
|
* Creates a new user on the system and assigns them access to the provided server.
|
|
* If the email address already belongs to a user on the system a new user will not
|
|
* be created.
|
|
*
|
|
* @throws \App\Exceptions\Model\DataValidationException
|
|
* @throws \App\Exceptions\Service\Subuser\ServerSubuserExistsException
|
|
* @throws \App\Exceptions\Service\Subuser\UserIsServerOwnerException
|
|
* @throws \Throwable
|
|
*/
|
|
public function handle(Server $server, string $email, array $permissions): Subuser
|
|
{
|
|
return $this->connection->transaction(function () use ($server, $email, $permissions) {
|
|
$user = User::query()->where('email', $email)->first();
|
|
if (!$user) {
|
|
// Just cap the username generated at 64 characters at most and then append a random string
|
|
// to the end to make it "unique"...
|
|
$username = substr(preg_replace('/([^\w\.-]+)/', '', strtok($email, '@')), 0, 64) . Str::random(3);
|
|
|
|
$user = $this->userCreationService->handle([
|
|
'email' => $email,
|
|
'username' => $username,
|
|
'name_first' => 'Server',
|
|
'name_last' => 'Subuser',
|
|
'root_admin' => false,
|
|
]);
|
|
}
|
|
|
|
if ($server->owner_id === $user->id) {
|
|
throw new UserIsServerOwnerException(trans('exceptions.subusers.user_is_owner'));
|
|
}
|
|
|
|
$subuserCount = $server->subusers()->where('user_id', $user->id)->count();
|
|
if ($subuserCount !== 0) {
|
|
throw new ServerSubuserExistsException(trans('exceptions.subusers.subuser_exists'));
|
|
}
|
|
|
|
$subuser = Subuser::query()->create([
|
|
'user_id' => $user->id,
|
|
'server_id' => $server->id,
|
|
'permissions' => array_unique($permissions),
|
|
]);
|
|
|
|
$subuser->user->notify(new AddedToServer([
|
|
'user' => $subuser->user->name_first,
|
|
'name' => $subuser->server->name,
|
|
'uuid_short' => $subuser->server->uuid_short,
|
|
]));
|
|
|
|
return $subuser;
|
|
});
|
|
}
|
|
}
|