mirror of
https://github.com/pelican-dev/panel.git
synced 2025-05-20 00:34:44 +02:00
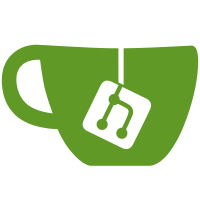
* Convert route options to fluent methods Laravel 8 adopts the tuple syntax for controller actions. Since the old options array is incompatible with this syntax, Shift converted them to use modern, fluent methods. * Slim `lang` files * Shift core files * Validate via object directly within Controllers * Use `Gate` facade for controller authorization * Dispatch jobs directly * Remove base controller inheritance * Default config files In an effort to make upgrading the constantly changing config files easier, Shift defaulted them and merged your true customizations - where ENV variables may not be used. * Set new `ENV` variables * Add new Laravel `composer run dev` script * Add `storage/app/private` folder * Bump Composer dependencies * Convert `$casts` property to method * Adopt Laravel type hints * Shift cleanup * Apply suggestions from code review Co-authored-by: MartinOscar <40749467+rmartinoscar@users.noreply.github.com> * Add old key as backup * Update composer * Remove extra line * Update this --------- Co-authored-by: Shift <shift@laravelshift.com> Co-authored-by: MartinOscar <40749467+rmartinoscar@users.noreply.github.com>
96 lines
2.3 KiB
PHP
96 lines
2.3 KiB
PHP
<?php
|
|
|
|
namespace App\Tests\Feature\Webhooks;
|
|
|
|
use App\Jobs\ProcessWebhook;
|
|
use App\Models\Server;
|
|
use App\Models\WebhookConfiguration;
|
|
use App\Tests\TestCase;
|
|
use Illuminate\Foundation\Testing\LazilyRefreshDatabase;
|
|
use Illuminate\Support\Facades\Queue;
|
|
|
|
class DispatchWebhooksTest extends TestCase
|
|
{
|
|
use LazilyRefreshDatabase;
|
|
|
|
protected function setUp(): void
|
|
{
|
|
parent::setUp();
|
|
Queue::fake();
|
|
}
|
|
|
|
public function test_it_sends_a_single_webhook(): void
|
|
{
|
|
WebhookConfiguration::factory()->create([
|
|
'events' => ['eloquent.created: '.Server::class],
|
|
]);
|
|
|
|
$this->createServer();
|
|
|
|
Queue::assertPushed(ProcessWebhook::class);
|
|
}
|
|
|
|
public function test_sends_multiple_webhooks(): void
|
|
{
|
|
WebhookConfiguration::factory(2)
|
|
->create(['events' => ['eloquent.created: '.Server::class]]);
|
|
|
|
$this->createServer();
|
|
|
|
Queue::assertPushed(ProcessWebhook::class, 2);
|
|
}
|
|
|
|
public function test_it_sends_no_webhooks(): void
|
|
{
|
|
WebhookConfiguration::factory()->create();
|
|
|
|
$this->createServer();
|
|
|
|
Queue::assertNothingPushed();
|
|
}
|
|
|
|
public function test_it_sends_some_webhooks(): void
|
|
{
|
|
WebhookConfiguration::factory(2)
|
|
->sequence(
|
|
['events' => ['eloquent.created: '.Server::class]],
|
|
['events' => ['eloquent.deleted: '.Server::class]]
|
|
)->create();
|
|
|
|
$this->createServer();
|
|
|
|
Queue::assertPushed(ProcessWebhook::class, 1);
|
|
}
|
|
|
|
public function test_it_does_not_call_removed_events(): void
|
|
{
|
|
$webhookConfig = WebhookConfiguration::factory()->create([
|
|
'events' => ['eloquent.created: '.Server::class],
|
|
]);
|
|
|
|
$webhookConfig->update(['events' => 'eloquent.deleted: '.Server::class]);
|
|
|
|
$this->createServer();
|
|
|
|
Queue::assertNothingPushed();
|
|
}
|
|
|
|
public function test_it_does_not_call_deleted_webhooks(): void
|
|
{
|
|
$webhookConfig = WebhookConfiguration::factory()->create([
|
|
'events' => ['eloquent.created: '.Server::class],
|
|
]);
|
|
|
|
$webhookConfig->delete();
|
|
|
|
$this->createServer();
|
|
|
|
Queue::assertNothingPushed();
|
|
}
|
|
|
|
public function createServer(): Server
|
|
{
|
|
return Server::factory()->withNode()->create();
|
|
}
|
|
}
|