mirror of
https://github.com/pelican-dev/panel.git
synced 2025-07-10 14:51:07 +02:00
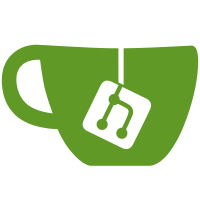
* add spatie health * change slug for health page * add check for panel version * only check for debug mode if env isn't local * add check for node versions * improve short summary * fix outdated check * run pint * fix health checks during tests * add count to ok message * fix typo * temp fix for phpstan job * fix pint... * improve "outdated" count Co-authored-by: MartinOscar <40749467+RMartinOscar@users.noreply.github.com> * run pint * skip node versions check if no nodes are created * auto run health checks if they didn't run before * small refactor * update navigation Co-authored-by: Charles <sir3lit@gmail.com> * fix errors if tests didn't run yet * fix disk usage check * remove plugin and use own page * use health status indicator from spatie * fix after merge * update icon * update color classes * fix after merge * add back imports oops... * wrong import oops²... * update spatie/laravel-health to latest * move Health page to correct namespace * update NodeVersionsCheck * use style instead of tailwind classes workaround until we have vite * cleanup custom checks --------- Co-authored-by: MartinOscar <40749467+RMartinOscar@users.noreply.github.com> Co-authored-by: Charles <sir3lit@gmail.com>
63 lines
2.3 KiB
PHP
63 lines
2.3 KiB
PHP
<?php
|
|
|
|
namespace App\Console;
|
|
|
|
use App\Console\Commands\Egg\CheckEggUpdatesCommand;
|
|
use App\Console\Commands\Maintenance\CleanServiceBackupFilesCommand;
|
|
use App\Console\Commands\Maintenance\PruneImagesCommand;
|
|
use App\Console\Commands\Maintenance\PruneOrphanedBackupsCommand;
|
|
use App\Console\Commands\Schedule\ProcessRunnableCommand;
|
|
use App\Jobs\NodeStatistics;
|
|
use App\Models\ActivityLog;
|
|
use App\Models\Webhook;
|
|
use Illuminate\Console\Scheduling\Schedule;
|
|
use Illuminate\Database\Console\PruneCommand;
|
|
use Illuminate\Foundation\Console\Kernel as ConsoleKernel;
|
|
use Spatie\Health\Commands\RunHealthChecksCommand;
|
|
use Spatie\Health\Commands\ScheduleCheckHeartbeatCommand;
|
|
|
|
class Kernel extends ConsoleKernel
|
|
{
|
|
/**
|
|
* Register the commands for the application.
|
|
*/
|
|
protected function commands(): void
|
|
{
|
|
$this->load(__DIR__ . '/Commands');
|
|
}
|
|
|
|
/**
|
|
* Define the application's command schedule.
|
|
*/
|
|
protected function schedule(Schedule $schedule): void
|
|
{
|
|
// https://laravel.com/docs/10.x/upgrade#redis-cache-tags
|
|
$schedule->command('cache:prune-stale-tags')->hourly();
|
|
|
|
// Execute scheduled commands for servers every minute, as if there was a normal cron running.
|
|
$schedule->command(ProcessRunnableCommand::class)->everyMinute()->withoutOverlapping();
|
|
|
|
$schedule->command(CleanServiceBackupFilesCommand::class)->daily();
|
|
$schedule->command(PruneImagesCommand::class)->daily();
|
|
$schedule->command(CheckEggUpdatesCommand::class)->hourly();
|
|
|
|
$schedule->job(new NodeStatistics())->everyFiveSeconds()->withoutOverlapping();
|
|
|
|
if (config('backups.prune_age')) {
|
|
// Every 30 minutes, run the backup pruning command so that any abandoned backups can be deleted.
|
|
$schedule->command(PruneOrphanedBackupsCommand::class)->everyThirtyMinutes();
|
|
}
|
|
|
|
if (config('activity.prune_days')) {
|
|
$schedule->command(PruneCommand::class, ['--model' => [ActivityLog::class]])->daily();
|
|
}
|
|
|
|
if (config('panel.webhook.prune_days')) {
|
|
$schedule->command(PruneCommand::class, ['--model' => [Webhook::class]])->daily();
|
|
}
|
|
|
|
$schedule->command(ScheduleCheckHeartbeatCommand::class)->everyMinute();
|
|
$schedule->command(RunHealthChecksCommand::class)->everyFiveMinutes();
|
|
}
|
|
}
|