mirror of
https://github.com/pelican-dev/panel.git
synced 2025-07-11 11:21:08 +02:00
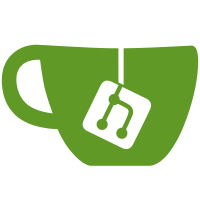
* force app timezone to be UTC * remove asDateTime overwrite * add custom column to display dates in user timezone * use `APP_TIMEZONE` as default timezone for new users * revert accidental pinting
75 lines
3.1 KiB
PHP
75 lines
3.1 KiB
PHP
<?php
|
|
|
|
namespace App\Filament\Resources\DatabaseHostResource\RelationManagers;
|
|
|
|
use App\Models\Database;
|
|
use App\Services\Databases\DatabasePasswordService;
|
|
use App\Tables\Columns\DateTimeColumn;
|
|
use Filament\Forms\Components\Actions\Action;
|
|
use Filament\Forms\Components\TextInput;
|
|
use Filament\Forms\Form;
|
|
use Filament\Forms\Get;
|
|
use Filament\Forms\Set;
|
|
use Filament\Resources\RelationManagers\RelationManager;
|
|
use Filament\Tables\Actions\DeleteAction;
|
|
use Filament\Tables\Actions\ViewAction;
|
|
use Filament\Tables\Columns\TextColumn;
|
|
use Filament\Tables\Table;
|
|
|
|
class DatabasesRelationManager extends RelationManager
|
|
{
|
|
protected static string $relationship = 'databases';
|
|
|
|
public function form(Form $form): Form
|
|
{
|
|
return $form
|
|
->schema([
|
|
TextInput::make('database')->columnSpanFull(),
|
|
TextInput::make('username'),
|
|
TextInput::make('password')
|
|
->hintAction(
|
|
Action::make('rotate')
|
|
->icon('tabler-refresh')
|
|
->requiresConfirmation()
|
|
->action(fn (DatabasePasswordService $service, Database $database, $set, $get) => $this->rotatePassword($service, $database, $set, $get))
|
|
)
|
|
->formatStateUsing(fn (Database $database) => $database->password),
|
|
TextInput::make('remote')->label('Connections From'),
|
|
TextInput::make('max_connections'),
|
|
TextInput::make('JDBC')
|
|
->label('JDBC Connection String')
|
|
->columnSpanFull()
|
|
->formatStateUsing(fn (Get $get, Database $database) => 'jdbc:mysql://' . $get('username') . ':' . urlencode($database->password) . '@' . $database->host->host . ':' . $database->host->port . '/' . $get('database')),
|
|
]);
|
|
}
|
|
|
|
public function table(Table $table): Table
|
|
{
|
|
return $table
|
|
->recordTitleAttribute('servers')
|
|
->columns([
|
|
TextColumn::make('database')->icon('tabler-database'),
|
|
TextColumn::make('username')->icon('tabler-user'),
|
|
TextColumn::make('remote'),
|
|
TextColumn::make('server.name')
|
|
->icon('tabler-brand-docker')
|
|
->url(fn (Database $database) => route('filament.admin.resources.servers.edit', ['record' => $database->server_id])),
|
|
TextColumn::make('max_connections'),
|
|
DateTimeColumn::make('created_at'),
|
|
])
|
|
->actions([
|
|
DeleteAction::make(),
|
|
ViewAction::make()->color('primary'),
|
|
]);
|
|
}
|
|
|
|
protected function rotatePassword(DatabasePasswordService $service, Database $database, Set $set, Get $get): void
|
|
{
|
|
$newPassword = $service->handle($database);
|
|
$jdbcString = 'jdbc:mysql://' . $get('username') . ':' . urlencode($newPassword) . '@' . $database->host->host . ':' . $database->host->port . '/' . $get('database');
|
|
|
|
$set('password', $newPassword);
|
|
$set('JDBC', $jdbcString);
|
|
}
|
|
}
|