mirror of
https://github.com/pelican-dev/panel.git
synced 2025-05-20 05:14:46 +02:00
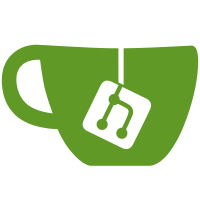
* add node cpu limit to backend * update makenodecommand * add node cpu limit to frontend * add migration and update mysql schema * run pint * fix typo in mysql schema * forgot this assert * forgot to setCpu here * run pint * adjust migration * Fix db migration * make cpu optional * set default value for cpu in node deployment * update mysql schema --------- Co-authored-by: notCharles <charles@pelican.dev>
51 lines
1.5 KiB
PHP
51 lines
1.5 KiB
PHP
<?php
|
|
|
|
namespace App\Tests\Integration\Services\Deployment;
|
|
|
|
use App\Models\Node;
|
|
use App\Models\Server;
|
|
use App\Models\Database;
|
|
use App\Tests\Integration\IntegrationTestCase;
|
|
use App\Services\Deployment\FindViableNodesService;
|
|
|
|
class FindViableNodesServiceTest extends IntegrationTestCase
|
|
{
|
|
protected function setUp(): void
|
|
{
|
|
parent::setUp();
|
|
|
|
Database::query()->delete();
|
|
Server::query()->delete();
|
|
Node::query()->delete();
|
|
}
|
|
|
|
public function testExceptionIsThrownIfNoMemoryHasBeenSet(): void
|
|
{
|
|
$this->expectException(\InvalidArgumentException::class);
|
|
$this->expectExceptionMessage('Memory usage must be an int, got NULL');
|
|
|
|
$this->getService()->setDisk(10)->setCpu(10)->handle();
|
|
}
|
|
|
|
public function testExceptionIsThrownIfNoDiskSpaceHasBeenSet(): void
|
|
{
|
|
$this->expectException(\InvalidArgumentException::class);
|
|
$this->expectExceptionMessage('Disk space must be an int, got NULL');
|
|
|
|
$this->getService()->setMemory(10)->setCpu(10)->handle();
|
|
}
|
|
|
|
public function testExceptionIsThrownIfNoCpuHasBeenSet(): void
|
|
{
|
|
$this->expectException(\InvalidArgumentException::class);
|
|
$this->expectExceptionMessage('CPU must be an int, got NULL');
|
|
|
|
$this->getService()->setMemory(10)->setDisk(10)->handle();
|
|
}
|
|
|
|
private function getService(): FindViableNodesService
|
|
{
|
|
return $this->app->make(FindViableNodesService::class);
|
|
}
|
|
}
|