mirror of
https://github.com/pelican-dev/panel.git
synced 2025-05-19 17:34:45 +02:00
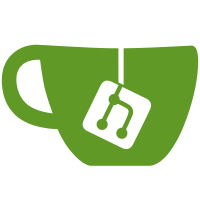
* chore: yarn upgrade * chore: composer upgrade * chore: php artisan filament:upgrade * chore: update filament-monaco-editor-views * chore: update filament-monaco-editor-configs * chore: move turnstile-views to plugins * fix monaco-editor loader & css
107 lines
3.6 KiB
PHP
107 lines
3.6 KiB
PHP
<?php
|
|
|
|
namespace App\Tests\Integration\Services\Backups;
|
|
|
|
use GuzzleHttp\Psr7\Request;
|
|
use GuzzleHttp\Psr7\Response;
|
|
use App\Models\Backup;
|
|
use GuzzleHttp\Exception\ClientException;
|
|
use App\Extensions\Backups\BackupManager;
|
|
use App\Extensions\Filesystem\S3Filesystem;
|
|
use App\Services\Backups\DeleteBackupService;
|
|
use App\Tests\Integration\IntegrationTestCase;
|
|
use App\Repositories\Daemon\DaemonBackupRepository;
|
|
use App\Exceptions\Service\Backup\BackupLockedException;
|
|
use Illuminate\Http\Client\ConnectionException;
|
|
|
|
class DeleteBackupServiceTest extends IntegrationTestCase
|
|
{
|
|
public function test_locked_backup_cannot_be_deleted(): void
|
|
{
|
|
$server = $this->createServerModel();
|
|
$backup = Backup::factory()->create([
|
|
'server_id' => $server->id,
|
|
'is_locked' => true,
|
|
]);
|
|
|
|
$this->expectException(BackupLockedException::class);
|
|
|
|
$this->app->make(DeleteBackupService::class)->handle($backup);
|
|
}
|
|
|
|
public function test_failed_backup_that_is_locked_can_be_deleted(): void
|
|
{
|
|
$server = $this->createServerModel();
|
|
$backup = Backup::factory()->create([
|
|
'server_id' => $server->id,
|
|
'is_locked' => true,
|
|
'is_successful' => false,
|
|
]);
|
|
|
|
$mock = $this->mock(DaemonBackupRepository::class);
|
|
$mock->expects('setServer->delete')->with($backup)->andReturn(new Response());
|
|
|
|
$this->app->make(DeleteBackupService::class)->handle($backup);
|
|
|
|
$backup->refresh();
|
|
|
|
$this->assertNotNull($backup->deleted_at);
|
|
}
|
|
|
|
public function test_exception_thrown_due_to_missing_backup_is_ignored(): void
|
|
{
|
|
$server = $this->createServerModel();
|
|
$backup = Backup::factory()->create(['server_id' => $server->id]);
|
|
|
|
$mock = $this->mock(DaemonBackupRepository::class);
|
|
$mock->expects('setServer->delete')->with($backup)->andThrow(new ConnectionException(previous: new ClientException('', new Request('DELETE', '/'), new Response(404))));
|
|
|
|
$this->app->make(DeleteBackupService::class)->handle($backup);
|
|
|
|
$backup->refresh();
|
|
|
|
$this->assertNotNull($backup->deleted_at);
|
|
}
|
|
|
|
public function test_exception_is_thrown_if_not404(): void
|
|
{
|
|
$server = $this->createServerModel();
|
|
$backup = Backup::factory()->create(['server_id' => $server->id]);
|
|
|
|
$mock = $this->mock(DaemonBackupRepository::class);
|
|
$mock->expects('setServer->delete')->with($backup)->andThrow(new ConnectionException(previous: new ClientException('', new Request('DELETE', '/'), new Response(500))));
|
|
|
|
$this->expectException(ConnectionException::class);
|
|
|
|
$this->app->make(DeleteBackupService::class)->handle($backup);
|
|
|
|
$backup->refresh();
|
|
|
|
$this->assertNull($backup->deleted_at);
|
|
}
|
|
|
|
public function test_s3_object_can_be_deleted(): void
|
|
{
|
|
$server = $this->createServerModel();
|
|
$backup = Backup::factory()->create([
|
|
'disk' => Backup::ADAPTER_AWS_S3,
|
|
'server_id' => $server->id,
|
|
]);
|
|
|
|
$manager = $this->mock(BackupManager::class);
|
|
$adapter = $this->mock(S3Filesystem::class);
|
|
|
|
$manager->expects('adapter')->with(Backup::ADAPTER_AWS_S3)->andReturn($adapter);
|
|
|
|
$adapter->expects('getBucket')->andReturn('foobar');
|
|
$adapter->expects('getClient->deleteObject')->with([
|
|
'Bucket' => 'foobar',
|
|
'Key' => sprintf('%s/%s.tar.gz', $server->uuid, $backup->uuid),
|
|
]);
|
|
|
|
$this->app->make(DeleteBackupService::class)->handle($backup);
|
|
|
|
$this->assertSoftDeleted($backup);
|
|
}
|
|
}
|