mirror of
https://github.com/pelican-dev/panel.git
synced 2025-05-19 17:34:45 +02:00
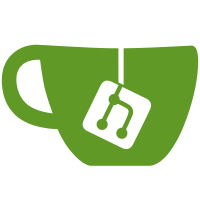
* chore: yarn upgrade * chore: composer upgrade * chore: php artisan filament:upgrade * chore: update filament-monaco-editor-views * chore: update filament-monaco-editor-configs * chore: move turnstile-views to plugins * fix monaco-editor loader & css
96 lines
2.3 KiB
PHP
96 lines
2.3 KiB
PHP
<?php
|
|
|
|
namespace App\Tests\Feature\Webhooks;
|
|
|
|
use App\Jobs\ProcessWebhook;
|
|
use App\Models\Server;
|
|
use App\Models\WebhookConfiguration;
|
|
use App\Tests\TestCase;
|
|
use Illuminate\Foundation\Testing\LazilyRefreshDatabase;
|
|
use Illuminate\Support\Facades\Queue;
|
|
|
|
class DispatchWebhooksTest extends TestCase
|
|
{
|
|
use LazilyRefreshDatabase;
|
|
|
|
protected function setUp(): void
|
|
{
|
|
parent::setUp();
|
|
Queue::fake();
|
|
}
|
|
|
|
public function test_it_sends_a_single_webhook(): void
|
|
{
|
|
WebhookConfiguration::factory()->create([
|
|
'events' => ['eloquent.created: '.Server::class],
|
|
]);
|
|
|
|
$this->createServer();
|
|
|
|
Queue::assertPushed(ProcessWebhook::class);
|
|
}
|
|
|
|
public function test_sends_multiple_webhooks()
|
|
{
|
|
WebhookConfiguration::factory(2)
|
|
->create(['events' => ['eloquent.created: '.Server::class]]);
|
|
|
|
$this->createServer();
|
|
|
|
Queue::assertPushed(ProcessWebhook::class, 2);
|
|
}
|
|
|
|
public function test_it_sends_no_webhooks()
|
|
{
|
|
WebhookConfiguration::factory()->create();
|
|
|
|
$this->createServer();
|
|
|
|
Queue::assertNothingPushed();
|
|
}
|
|
|
|
public function test_it_sends_some_webhooks()
|
|
{
|
|
WebhookConfiguration::factory(2)
|
|
->sequence(
|
|
['events' => ['eloquent.created: '.Server::class]],
|
|
['events' => ['eloquent.deleted: '.Server::class]]
|
|
)->create();
|
|
|
|
$this->createServer();
|
|
|
|
Queue::assertPushed(ProcessWebhook::class, 1);
|
|
}
|
|
|
|
public function test_it_does_not_call_removed_events()
|
|
{
|
|
$webhookConfig = WebhookConfiguration::factory()->create([
|
|
'events' => ['eloquent.created: '.Server::class],
|
|
]);
|
|
|
|
$webhookConfig->update(['events' => 'eloquent.deleted: '.Server::class]);
|
|
|
|
$this->createServer();
|
|
|
|
Queue::assertNothingPushed();
|
|
}
|
|
|
|
public function test_it_does_not_call_deleted_webhooks()
|
|
{
|
|
$webhookConfig = WebhookConfiguration::factory()->create([
|
|
'events' => ['eloquent.created: '.Server::class],
|
|
]);
|
|
|
|
$webhookConfig->delete();
|
|
|
|
$this->createServer();
|
|
|
|
Queue::assertNothingPushed();
|
|
}
|
|
|
|
public function createServer(): Server
|
|
{
|
|
return Server::factory()->withNode()->create();
|
|
}
|
|
}
|