mirror of
https://github.com/pelican-dev/panel.git
synced 2025-05-20 01:44:45 +02:00
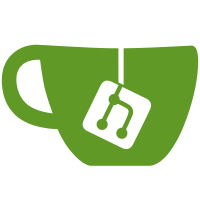
* Remove open in new tab since both are on filament now. Removing the open in new tab since both are on filament now. And the tenant: null was function default so not needed aswell * Rework permission tab loading Reworked permission tab loading to make it easier to expand on it in the future. This is way more friendly if extensions are planned in the future. * Rework permission tab loading Reworked permission tab loading to make it easier to expand on it in the future. This is way more friendly if extensions are planned in the future. * Rework permission tab loading Reworked permission tab loading to make it easier to expand on it in the future. This is way more friendly if extensions are planned in the future. * Update UserResource.php Used wrong name. It's not the name, the label has to be checked there. * Fix: wrong name used Used wrong name. It's not the name, the label has to be checked there. * Update permission loading Moved permission list to app/Models/Permission.php and made UserResource.php and ListUsers.php use it. * Fix Pint and PHPStan error Added comments * Update array key Updated array key using the lowercase name. Suggested by https://github.com/Boy132 * Correct array key Updated array key using the lowercase. Suggested by https://github.com/Boy132 * Revert/correct array key Updated array key using the lowercase and the correct label. * Add 'user' key In the old $permission array was user an entry witch is missing in permissionTabs() * Style and return Added @return and removed empty lines * pin fix pint * fix pint remove @return * fix pint add () since pint is still not happy * remove mb_strtolower mb_strtolower is not necessary * remove schema for control remove ->schema for control tab. * Remove import Remove unused import * correct translation key Co-authored-by: Boy132 <Boy132@users.noreply.github.com> * make columns optional, checkboxList => columns is now optional and default to 2 * move user and control registration removed control registration since it was duplicate and move user registration to permissionTabs * update @return on permissionTabs() * Fix array key warning * simplify permissions data * revert this * fix edit modal * update icons --------- Co-authored-by: Boy132 <Boy132@users.noreply.github.com> Co-authored-by: Boy132 <mail@boy132.de>
213 lines
6.5 KiB
PHP
213 lines
6.5 KiB
PHP
<?php
|
|
|
|
namespace App\Models;
|
|
|
|
use App\Contracts\Validatable;
|
|
use App\Traits\HasValidation;
|
|
use Illuminate\Database\Eloquent\Factories\HasFactory;
|
|
use Illuminate\Database\Eloquent\Model;
|
|
use Illuminate\Support\Collection;
|
|
|
|
class Permission extends Model implements Validatable
|
|
{
|
|
use HasFactory, HasValidation;
|
|
|
|
/**
|
|
* The resource name for this model when it is transformed into an
|
|
* API representation using fractal.
|
|
*/
|
|
public const RESOURCE_NAME = 'subuser_permission';
|
|
|
|
/**
|
|
* Constants defining different permissions available.
|
|
*/
|
|
public const ACTION_WEBSOCKET_CONNECT = 'websocket.connect';
|
|
|
|
public const ACTION_CONTROL_CONSOLE = 'control.console';
|
|
|
|
public const ACTION_CONTROL_START = 'control.start';
|
|
|
|
public const ACTION_CONTROL_STOP = 'control.stop';
|
|
|
|
public const ACTION_CONTROL_RESTART = 'control.restart';
|
|
|
|
public const ACTION_DATABASE_READ = 'database.read';
|
|
|
|
public const ACTION_DATABASE_CREATE = 'database.create';
|
|
|
|
public const ACTION_DATABASE_UPDATE = 'database.update';
|
|
|
|
public const ACTION_DATABASE_DELETE = 'database.delete';
|
|
|
|
public const ACTION_DATABASE_VIEW_PASSWORD = 'database.view-password';
|
|
|
|
public const ACTION_SCHEDULE_READ = 'schedule.read';
|
|
|
|
public const ACTION_SCHEDULE_CREATE = 'schedule.create';
|
|
|
|
public const ACTION_SCHEDULE_UPDATE = 'schedule.update';
|
|
|
|
public const ACTION_SCHEDULE_DELETE = 'schedule.delete';
|
|
|
|
public const ACTION_USER_READ = 'user.read';
|
|
|
|
public const ACTION_USER_CREATE = 'user.create';
|
|
|
|
public const ACTION_USER_UPDATE = 'user.update';
|
|
|
|
public const ACTION_USER_DELETE = 'user.delete';
|
|
|
|
public const ACTION_BACKUP_READ = 'backup.read';
|
|
|
|
public const ACTION_BACKUP_CREATE = 'backup.create';
|
|
|
|
public const ACTION_BACKUP_DELETE = 'backup.delete';
|
|
|
|
public const ACTION_BACKUP_DOWNLOAD = 'backup.download';
|
|
|
|
public const ACTION_BACKUP_RESTORE = 'backup.restore';
|
|
|
|
public const ACTION_ALLOCATION_READ = 'allocation.read';
|
|
|
|
public const ACTION_ALLOCATION_CREATE = 'allocation.create';
|
|
|
|
public const ACTION_ALLOCATION_UPDATE = 'allocation.update';
|
|
|
|
public const ACTION_ALLOCATION_DELETE = 'allocation.delete';
|
|
|
|
public const ACTION_FILE_READ = 'file.read';
|
|
|
|
public const ACTION_FILE_READ_CONTENT = 'file.read-content';
|
|
|
|
public const ACTION_FILE_CREATE = 'file.create';
|
|
|
|
public const ACTION_FILE_UPDATE = 'file.update';
|
|
|
|
public const ACTION_FILE_DELETE = 'file.delete';
|
|
|
|
public const ACTION_FILE_ARCHIVE = 'file.archive';
|
|
|
|
public const ACTION_FILE_SFTP = 'file.sftp';
|
|
|
|
public const ACTION_STARTUP_READ = 'startup.read';
|
|
|
|
public const ACTION_STARTUP_UPDATE = 'startup.update';
|
|
|
|
public const ACTION_STARTUP_DOCKER_IMAGE = 'startup.docker-image';
|
|
|
|
public const ACTION_SETTINGS_RENAME = 'settings.rename';
|
|
|
|
public const ACTION_SETTINGS_REINSTALL = 'settings.reinstall';
|
|
|
|
public const ACTION_ACTIVITY_READ = 'activity.read';
|
|
|
|
public $timestamps = false;
|
|
|
|
/**
|
|
* Fields that are not mass assignable.
|
|
*/
|
|
protected $guarded = ['id', 'created_at', 'updated_at'];
|
|
|
|
/** @var array<array-key, string[]> */
|
|
public static array $validationRules = [
|
|
'subuser_id' => ['required', 'numeric', 'min:1'],
|
|
'permission' => ['required', 'string'],
|
|
];
|
|
|
|
protected function casts(): array
|
|
{
|
|
return [
|
|
'subuser_id' => 'integer',
|
|
];
|
|
}
|
|
|
|
/**
|
|
* All the permissions available on the system.
|
|
*
|
|
* @return array<int, array{
|
|
* name: string,
|
|
* icon: string,
|
|
* permissions: string[]
|
|
* }>
|
|
*/
|
|
public static function permissionData(): array
|
|
{
|
|
return [
|
|
[
|
|
'name' => 'control',
|
|
'icon' => 'tabler-terminal-2',
|
|
'permissions' => ['console', 'start', 'stop', 'restart'],
|
|
],
|
|
[
|
|
'name' => 'user',
|
|
'icon' => 'tabler-users',
|
|
'permissions' => ['read', 'create', 'update', 'delete'],
|
|
],
|
|
[
|
|
'name' => 'file',
|
|
'icon' => 'tabler-files',
|
|
'permissions' => ['read', 'read-content', 'create', 'update', 'delete', 'archive', 'sftp'],
|
|
],
|
|
[
|
|
'name' => 'backup',
|
|
'icon' => 'tabler-file-zip',
|
|
'permissions' => ['read', 'create', 'delete', 'download', 'restore'],
|
|
],
|
|
[
|
|
'name' => 'allocation',
|
|
'icon' => 'tabler-network',
|
|
'permissions' => ['read', 'create', 'update', 'delete'],
|
|
],
|
|
[
|
|
'name' => 'startup',
|
|
'icon' => 'tabler-player-play',
|
|
'permissions' => ['read', 'update', 'docker-image'],
|
|
],
|
|
[
|
|
'name' => 'database',
|
|
'icon' => 'tabler-database',
|
|
'permissions' => ['read', 'create', 'update', 'delete', 'view-password'],
|
|
],
|
|
[
|
|
'name' => 'schedule',
|
|
'icon' => 'tabler-clock',
|
|
'permissions' => ['read', 'create', 'update', 'delete'],
|
|
],
|
|
[
|
|
'name' => 'settings',
|
|
'icon' => 'tabler-settings',
|
|
'permissions' => ['rename', 'reinstall'],
|
|
],
|
|
[
|
|
'name' => 'activity',
|
|
'icon' => 'tabler-stack',
|
|
'permissions' => ['read'],
|
|
],
|
|
];
|
|
}
|
|
|
|
/**
|
|
* Returns all the permissions available on the system for a user to have when controlling a server.
|
|
*/
|
|
public static function permissions(): Collection
|
|
{
|
|
$permissions = [
|
|
'websocket' => [
|
|
'description' => 'Allows the user to connect to the server websocket, giving them access to view console output and realtime server stats.',
|
|
'keys' => [
|
|
'connect' => 'Allows a user to connect to the websocket instance for a server to stream the console.',
|
|
],
|
|
],
|
|
];
|
|
|
|
foreach (static::permissionData() as $data) {
|
|
$permissions[$data['name']] = [
|
|
'description' => trans('server/users.permissions.' . $data['name'] . '_desc'),
|
|
'keys' => collect($data['permissions'])->mapWithKeys(fn ($key) => [$key => trans('server/users.permissions.' . $data['name'] . '_' . str($key)->replace('-', '_'))])->toArray(),
|
|
];
|
|
}
|
|
|
|
return collect($permissions);
|
|
}
|
|
}
|