mirror of
https://github.com/pelican-dev/panel.git
synced 2025-07-04 02:11:08 +02:00
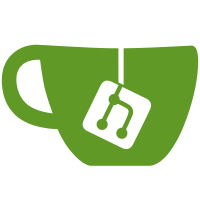
* Fix copy paste AllocationsRelationManager * We shouldn't let the user know if the user is correct but the password isn't * Add missing `trans()` `EditServer` * Add missing `trans()` User `ServersRelationManager` * Replace every `__()` with `trans()` helper * Fix `exceptions` `User` Model * Replace `Translator->get()` with `trans()` helper * Revert "We shouldn't let the user know if the user is correct but the password isn't" This reverts commit e156ee4b38e9e969662a532648c78fdc1e9b0166. that's stock laravel, therefore it needs to stay
429 lines
14 KiB
PHP
429 lines
14 KiB
PHP
<?php
|
|
|
|
namespace App\Models;
|
|
|
|
use App\Contracts\Validatable;
|
|
use App\Exceptions\DisplayException;
|
|
use App\Rules\Username;
|
|
use App\Facades\Activity;
|
|
use App\Traits\HasValidation;
|
|
use DateTimeZone;
|
|
use Filament\Models\Contracts\FilamentUser;
|
|
use Filament\Models\Contracts\HasAvatar;
|
|
use Filament\Models\Contracts\HasName;
|
|
use Filament\Models\Contracts\HasTenants;
|
|
use Filament\Panel;
|
|
use Illuminate\Database\Eloquent\Factories\HasFactory;
|
|
use Illuminate\Database\Eloquent\Model;
|
|
use Illuminate\Database\Eloquent\Relations\BelongsToMany;
|
|
use Illuminate\Support\Collection;
|
|
use Illuminate\Support\Str;
|
|
use Illuminate\Validation\Rules\In;
|
|
use Illuminate\Auth\Authenticatable;
|
|
use Illuminate\Notifications\Notifiable;
|
|
use Illuminate\Database\Eloquent\Builder;
|
|
use App\Models\Traits\HasAccessTokens;
|
|
use Illuminate\Auth\Passwords\CanResetPassword;
|
|
use Illuminate\Database\Eloquent\Relations\HasMany;
|
|
use Illuminate\Foundation\Auth\Access\Authorizable;
|
|
use Illuminate\Database\Eloquent\Relations\MorphToMany;
|
|
use Illuminate\Contracts\Auth\Authenticatable as AuthenticatableContract;
|
|
use Illuminate\Contracts\Auth\Access\Authorizable as AuthorizableContract;
|
|
use Illuminate\Contracts\Auth\CanResetPassword as CanResetPasswordContract;
|
|
use App\Notifications\SendPasswordReset as ResetPasswordNotification;
|
|
use Filament\Facades\Filament;
|
|
use ResourceBundle;
|
|
use Spatie\Permission\Traits\HasRoles;
|
|
|
|
/**
|
|
* App\Models\User.
|
|
*
|
|
* @property int $id
|
|
* @property string|null $external_id
|
|
* @property string $uuid
|
|
* @property string $username
|
|
* @property string $email
|
|
* @property string $password
|
|
* @property string|null $remember_token
|
|
* @property string $language
|
|
* @property string $timezone
|
|
* @property bool $use_totp
|
|
* @property string|null $totp_secret
|
|
* @property \Illuminate\Support\Carbon|null $totp_authenticated_at
|
|
* @property array|null $oauth
|
|
* @property bool $gravatar
|
|
* @property \Illuminate\Support\Carbon|null $created_at
|
|
* @property \Illuminate\Support\Carbon|null $updated_at
|
|
* @property \Illuminate\Database\Eloquent\Collection|\App\Models\ApiKey[] $apiKeys
|
|
* @property int|null $api_keys_count
|
|
* @property string $name
|
|
* @property \Illuminate\Notifications\DatabaseNotificationCollection|\Illuminate\Notifications\DatabaseNotification[] $notifications
|
|
* @property int|null $notifications_count
|
|
* @property \Illuminate\Database\Eloquent\Collection|\App\Models\RecoveryToken[] $recoveryTokens
|
|
* @property int|null $recovery_tokens_count
|
|
* @property \Illuminate\Database\Eloquent\Collection|\App\Models\Server[] $servers
|
|
* @property int|null $servers_count
|
|
* @property \Illuminate\Database\Eloquent\Collection|\App\Models\UserSSHKey[] $sshKeys
|
|
* @property int|null $ssh_keys_count
|
|
* @property \Illuminate\Database\Eloquent\Collection|\App\Models\ApiKey[] $tokens
|
|
* @property int|null $tokens_count
|
|
*
|
|
* @method static \Database\Factories\UserFactory factory(...$parameters)
|
|
* @method static Builder|User newModelQuery()
|
|
* @method static Builder|User newQuery()
|
|
* @method static Builder|User query()
|
|
* @method static Builder|User whereCreatedAt($value)
|
|
* @method static Builder|User whereEmail($value)
|
|
* @method static Builder|User whereExternalId($value)
|
|
* @method static Builder|User whereGravatar($value)
|
|
* @method static Builder|User whereId($value)
|
|
* @method static Builder|User whereLanguage($value)
|
|
* @method static Builder|User whereTimezone($value)
|
|
* @method static Builder|User wherePassword($value)
|
|
* @method static Builder|User whereRememberToken($value)
|
|
* @method static Builder|User whereTotpAuthenticatedAt($value)
|
|
* @method static Builder|User whereTotpSecret($value)
|
|
* @method static Builder|User whereUpdatedAt($value)
|
|
* @method static Builder|User whereUseTotp($value)
|
|
* @method static Builder|User whereUsername($value)
|
|
* @method static Builder|User whereUuid($value)
|
|
*/
|
|
class User extends Model implements AuthenticatableContract, AuthorizableContract, CanResetPasswordContract, FilamentUser, HasAvatar, HasName, HasTenants, Validatable
|
|
{
|
|
use Authenticatable;
|
|
use Authorizable { can as protected canned; }
|
|
use CanResetPassword;
|
|
use HasAccessTokens;
|
|
use HasFactory;
|
|
use HasRoles;
|
|
use HasValidation { getRules as getValidationRules; }
|
|
use Notifiable;
|
|
|
|
public const USER_LEVEL_USER = 0;
|
|
|
|
public const USER_LEVEL_ADMIN = 1;
|
|
|
|
/**
|
|
* The resource name for this model when it is transformed into an
|
|
* API representation using fractal. Also used as name for api key permissions.
|
|
*/
|
|
public const RESOURCE_NAME = 'user';
|
|
|
|
/**
|
|
* A list of mass-assignable variables.
|
|
*/
|
|
protected $fillable = [
|
|
'external_id',
|
|
'username',
|
|
'email',
|
|
'password',
|
|
'language',
|
|
'timezone',
|
|
'use_totp',
|
|
'totp_secret',
|
|
'totp_authenticated_at',
|
|
'gravatar',
|
|
'oauth',
|
|
];
|
|
|
|
/**
|
|
* The attributes excluded from the model's JSON form.
|
|
*/
|
|
protected $hidden = ['password', 'remember_token', 'totp_secret', 'totp_authenticated_at', 'oauth'];
|
|
|
|
/**
|
|
* Default values for specific fields in the database.
|
|
*/
|
|
protected $attributes = [
|
|
'external_id' => null,
|
|
'language' => 'en',
|
|
'timezone' => 'UTC',
|
|
'use_totp' => false,
|
|
'totp_secret' => null,
|
|
'oauth' => '[]',
|
|
];
|
|
|
|
/**
|
|
* Rules verifying that the data being stored matches the expectations of the database.
|
|
*/
|
|
public static array $validationRules = [
|
|
'uuid' => 'nullable|string|size:36|unique:users,uuid',
|
|
'email' => 'required|email|between:1,255|unique:users,email',
|
|
'external_id' => 'sometimes|nullable|string|max:255|unique:users,external_id',
|
|
'username' => 'required|between:1,255|unique:users,username',
|
|
'password' => 'sometimes|nullable|string',
|
|
'language' => 'string',
|
|
'timezone' => 'string',
|
|
'use_totp' => 'boolean',
|
|
'totp_secret' => 'nullable|string',
|
|
'oauth' => 'array|nullable',
|
|
];
|
|
|
|
protected function casts(): array
|
|
{
|
|
return [
|
|
'use_totp' => 'boolean',
|
|
'gravatar' => 'boolean',
|
|
'totp_authenticated_at' => 'datetime',
|
|
'totp_secret' => 'encrypted',
|
|
'oauth' => 'array',
|
|
];
|
|
}
|
|
|
|
protected static function booted(): void
|
|
{
|
|
static::creating(function (self $user) {
|
|
$user->uuid ??= Str::uuid()->toString();
|
|
$user->timezone ??= config('app.timezone');
|
|
|
|
return true;
|
|
});
|
|
|
|
static::deleting(function (self $user) {
|
|
throw_if($user->servers()->count() > 0, new DisplayException(trans('exceptions.users.has_servers')));
|
|
|
|
throw_if(request()->user()?->id === $user->id, new DisplayException(trans('exceptions.users.is_self')));
|
|
});
|
|
}
|
|
|
|
/**
|
|
* Implement language verification by overriding Eloquence's gather
|
|
* rules function.
|
|
*/
|
|
public static function getRules(): array
|
|
{
|
|
$rules = self::getValidationRules();
|
|
|
|
$rules['language'][] = new In(array_values(array_filter(ResourceBundle::getLocales(''), fn ($lang) => preg_match('/^[a-z]{2}$/', $lang))));
|
|
$rules['timezone'][] = new In(DateTimeZone::listIdentifiers());
|
|
$rules['username'][] = new Username();
|
|
|
|
return $rules;
|
|
}
|
|
|
|
/**
|
|
* Return the user model in a format that can be passed over to React templates.
|
|
*/
|
|
public function toReactObject(): array
|
|
{
|
|
return array_merge(collect($this->toArray())->except(['id', 'external_id'])->toArray(), [
|
|
'root_admin' => $this->isRootAdmin(),
|
|
'admin' => $this->canAccessPanel(Filament::getPanel('admin')),
|
|
]);
|
|
}
|
|
|
|
/**
|
|
* Send the password reset notification.
|
|
*
|
|
* @param string $token
|
|
*/
|
|
public function sendPasswordResetNotification($token): void
|
|
{
|
|
Activity::event('auth:reset-password')
|
|
->withRequestMetadata()
|
|
->subject($this)
|
|
->log('sending password reset email');
|
|
|
|
$this->notify(new ResetPasswordNotification($token));
|
|
}
|
|
|
|
/**
|
|
* Store the username as a lowercase string.
|
|
*/
|
|
public function setUsernameAttribute(string $value): void
|
|
{
|
|
$this->attributes['username'] = mb_strtolower($value);
|
|
}
|
|
|
|
/**
|
|
* Store the email as a lowercase string.
|
|
*/
|
|
public function setEmailAttribute(string $value): void
|
|
{
|
|
$this->attributes['email'] = mb_strtolower($value);
|
|
}
|
|
|
|
/**
|
|
* Returns all servers that a user owns.
|
|
*
|
|
* @return HasMany<Server, $this>
|
|
*/
|
|
public function servers(): HasMany
|
|
{
|
|
return $this->hasMany(Server::class, 'owner_id');
|
|
}
|
|
|
|
public function apiKeys(): HasMany
|
|
{
|
|
return $this->hasMany(ApiKey::class)
|
|
->where('key_type', ApiKey::TYPE_ACCOUNT);
|
|
}
|
|
|
|
public function recoveryTokens(): HasMany
|
|
{
|
|
return $this->hasMany(RecoveryToken::class);
|
|
}
|
|
|
|
public function sshKeys(): HasMany
|
|
{
|
|
return $this->hasMany(UserSSHKey::class);
|
|
}
|
|
|
|
/**
|
|
* Returns all the activity logs where this user is the subject — not to
|
|
* be confused by activity logs where this user is the _actor_.
|
|
*/
|
|
public function activity(): MorphToMany
|
|
{
|
|
return $this->morphToMany(ActivityLog::class, 'subject', 'activity_log_subjects');
|
|
}
|
|
|
|
/**
|
|
* Returns all the servers that a user can access.
|
|
* Either because they are an admin or because they are the owner/ a subuser of the server.
|
|
*/
|
|
public function accessibleServers(): Builder
|
|
{
|
|
if ($this->canned('viewList server')) {
|
|
return Server::query();
|
|
}
|
|
|
|
return $this->directAccessibleServers();
|
|
}
|
|
|
|
/**
|
|
* Returns all the servers that a user can access "directly".
|
|
* This means either because they are the owner or a subuser of the server.
|
|
*/
|
|
public function directAccessibleServers(): Builder
|
|
{
|
|
return Server::query()
|
|
->select('servers.*')
|
|
->leftJoin('subusers', 'subusers.server_id', '=', 'servers.id')
|
|
->where(function (Builder $builder) {
|
|
$builder->where('servers.owner_id', $this->id)->orWhere('subusers.user_id', $this->id);
|
|
})
|
|
->groupBy('servers.id');
|
|
}
|
|
|
|
public function subusers(): HasMany
|
|
{
|
|
return $this->hasMany(Subuser::class);
|
|
}
|
|
|
|
public function subServers(): BelongsToMany
|
|
{
|
|
return $this->belongsToMany(Server::class, 'subusers');
|
|
}
|
|
|
|
protected function checkPermission(Server $server, string $permission = ''): bool
|
|
{
|
|
if ($this->canned('update server', $server) || $server->owner_id === $this->id) {
|
|
return true;
|
|
}
|
|
|
|
// If the user only has "view" permissions allow viewing the console
|
|
if ($permission === Permission::ACTION_WEBSOCKET_CONNECT && $this->canned('view server', $server)) {
|
|
return true;
|
|
}
|
|
|
|
$subuser = $server->subusers->where('user_id', $this->id)->first();
|
|
if (!$subuser || empty($permission)) {
|
|
return false;
|
|
}
|
|
|
|
$check = in_array($permission, $subuser->permissions);
|
|
|
|
return $check;
|
|
}
|
|
|
|
/**
|
|
* Laravel's policies strictly check for the existence of a real method,
|
|
* this checks if the ability is one of our permissions and then checks if the user can do it or not
|
|
* Otherwise it calls the Authorizable trait's parent method
|
|
*/
|
|
public function can($abilities, mixed $arguments = []): bool
|
|
{
|
|
if (is_string($abilities) && str_contains($abilities, '.')) {
|
|
[$permission, $key] = str($abilities)->explode('.', 2);
|
|
|
|
if (isset(Permission::permissions()[$permission]['keys'][$key])) {
|
|
if ($arguments instanceof Server) {
|
|
return $this->checkPermission($arguments, $abilities);
|
|
}
|
|
}
|
|
}
|
|
|
|
return $this->canned($abilities, $arguments);
|
|
}
|
|
|
|
public function isLastRootAdmin(): bool
|
|
{
|
|
$rootAdmins = User::all()->filter(fn ($user) => $user->isRootAdmin());
|
|
|
|
return once(fn () => $rootAdmins->count() === 1 && $rootAdmins->first()->is($this));
|
|
}
|
|
|
|
public function isRootAdmin(): bool
|
|
{
|
|
return $this->hasRole(Role::ROOT_ADMIN);
|
|
}
|
|
|
|
public function isAdmin(): bool
|
|
{
|
|
return $this->isRootAdmin() || ($this->roles()->count() >= 1 && $this->getAllPermissions()->count() >= 1);
|
|
}
|
|
|
|
public function canAccessPanel(Panel $panel): bool
|
|
{
|
|
if ($this->isRootAdmin()) {
|
|
return true;
|
|
}
|
|
|
|
if ($panel->getId() === 'admin') {
|
|
return $this->isAdmin();
|
|
}
|
|
|
|
return true;
|
|
}
|
|
|
|
public function getFilamentName(): string
|
|
{
|
|
return $this->username;
|
|
}
|
|
|
|
public function getFilamentAvatarUrl(): ?string
|
|
{
|
|
return 'https://gravatar.com/avatar/' . md5(strtolower($this->email));
|
|
}
|
|
|
|
public function canTarget(Model $user): bool
|
|
{
|
|
if ($this->isRootAdmin()) {
|
|
return true;
|
|
}
|
|
|
|
return $user instanceof User && !$user->isRootAdmin();
|
|
}
|
|
|
|
public function getTenants(Panel $panel): array|Collection
|
|
{
|
|
return $this->accessibleServers()->get();
|
|
}
|
|
|
|
public function canAccessTenant(Model $tenant): bool
|
|
{
|
|
if ($tenant instanceof Server) {
|
|
if ($this->canned('view server', $tenant) || $tenant->owner_id === $this->id) {
|
|
return true;
|
|
}
|
|
|
|
$subuser = $tenant->subusers->where('user_id', $this->id)->first();
|
|
|
|
return $subuser !== null;
|
|
}
|
|
|
|
return false;
|
|
}
|
|
}
|