mirror of
https://github.com/pelican-dev/panel.git
synced 2025-07-03 04:51:07 +02:00
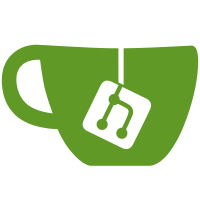
* add spatie health * change slug for health page * add check for panel version * only check for debug mode if env isn't local * add check for node versions * improve short summary * fix outdated check * run pint * fix health checks during tests * add count to ok message * fix typo * temp fix for phpstan job * fix pint... * improve "outdated" count Co-authored-by: MartinOscar <40749467+RMartinOscar@users.noreply.github.com> * run pint * skip node versions check if no nodes are created * auto run health checks if they didn't run before * small refactor * update navigation Co-authored-by: Charles <sir3lit@gmail.com> * fix errors if tests didn't run yet * fix disk usage check * remove plugin and use own page * use health status indicator from spatie * fix after merge * update icon * update color classes * fix after merge * add back imports oops... * wrong import oops²... * update spatie/laravel-health to latest * move Health page to correct namespace * update NodeVersionsCheck * use style instead of tailwind classes workaround until we have vite * cleanup custom checks --------- Co-authored-by: MartinOscar <40749467+RMartinOscar@users.noreply.github.com> Co-authored-by: Charles <sir3lit@gmail.com>
153 lines
5.8 KiB
PHP
153 lines
5.8 KiB
PHP
<?php
|
|
|
|
namespace App\Providers;
|
|
|
|
use App\Checks\NodeVersionsCheck;
|
|
use App\Checks\PanelVersionCheck;
|
|
use App\Checks\UsedDiskSpaceCheck;
|
|
use App\Filament\Server\Pages\Console;
|
|
use App\Models;
|
|
use App\Models\ApiKey;
|
|
use App\Models\Node;
|
|
use App\Models\User;
|
|
use App\Services\Helpers\SoftwareVersionService;
|
|
use Dedoc\Scramble\Scramble;
|
|
use Dedoc\Scramble\Support\Generator\OpenApi;
|
|
use Dedoc\Scramble\Support\Generator\SecurityScheme;
|
|
use Filament\Support\Colors\Color;
|
|
use Filament\Support\Facades\FilamentColor;
|
|
use Filament\Support\Facades\FilamentView;
|
|
use Filament\View\PanelsRenderHook;
|
|
use Illuminate\Database\Eloquent\Relations\Relation;
|
|
use Illuminate\Foundation\Application;
|
|
use Illuminate\Foundation\Console\AboutCommand;
|
|
use Illuminate\Support\Facades\Event;
|
|
use Illuminate\Support\Facades\Gate;
|
|
use Illuminate\Support\Facades\Http;
|
|
use Illuminate\Support\Facades\URL;
|
|
use Illuminate\Support\ServiceProvider;
|
|
use Illuminate\Support\Str;
|
|
use Laravel\Sanctum\Sanctum;
|
|
use SocialiteProviders\Manager\SocialiteWasCalled;
|
|
use Spatie\Health\Checks\Checks\CacheCheck;
|
|
use Spatie\Health\Checks\Checks\DatabaseCheck;
|
|
use Spatie\Health\Checks\Checks\DebugModeCheck;
|
|
use Spatie\Health\Checks\Checks\EnvironmentCheck;
|
|
use Spatie\Health\Checks\Checks\ScheduleCheck;
|
|
use Spatie\Health\Facades\Health;
|
|
|
|
class AppServiceProvider extends ServiceProvider
|
|
{
|
|
/**
|
|
* Bootstrap any application services.
|
|
*/
|
|
public function boot(Application $app, SoftwareVersionService $versionService): void
|
|
{
|
|
// If the APP_URL value is set with https:// make sure we force it here. Theoretically
|
|
// this should just work with the proxy logic, but there are a lot of cases where it
|
|
// doesn't, and it triggers a lot of support requests, so lets just head it off here.
|
|
URL::forceHttps(Str::startsWith(config('app.url') ?? '', 'https://'));
|
|
|
|
Relation::enforceMorphMap([
|
|
'allocation' => Models\Allocation::class,
|
|
'api_key' => Models\ApiKey::class,
|
|
'backup' => Models\Backup::class,
|
|
'database' => Models\Database::class,
|
|
'egg' => Models\Egg::class,
|
|
'egg_variable' => Models\EggVariable::class,
|
|
'schedule' => Models\Schedule::class,
|
|
'server' => Models\Server::class,
|
|
'ssh_key' => Models\UserSSHKey::class,
|
|
'task' => Models\Task::class,
|
|
'user' => Models\User::class,
|
|
]);
|
|
|
|
Http::macro(
|
|
'daemon',
|
|
fn (Node $node, array $headers = []) => Http::acceptJson()
|
|
->asJson()
|
|
->withToken($node->daemon_token)
|
|
->withHeaders($headers)
|
|
->withOptions(['verify' => (bool) $app->environment('production')])
|
|
->timeout(config('panel.guzzle.timeout'))
|
|
->connectTimeout(config('panel.guzzle.connect_timeout'))
|
|
->baseUrl($node->getConnectionAddress())
|
|
);
|
|
|
|
Sanctum::usePersonalAccessTokenModel(ApiKey::class);
|
|
|
|
$bearerTokens = fn (OpenApi $openApi) => $openApi->secure(SecurityScheme::http('bearer'));
|
|
Gate::define('viewApiDocs', fn () => true);
|
|
Scramble::registerApi('application', ['api_path' => 'api/application', 'info' => ['version' => '1.0']]);
|
|
Scramble::registerApi('client', ['api_path' => 'api/client', 'info' => ['version' => '1.0']])->afterOpenApiGenerated($bearerTokens);
|
|
Scramble::registerApi('remote', ['api_path' => 'api/remote', 'info' => ['version' => '1.0']])->afterOpenApiGenerated($bearerTokens);
|
|
|
|
$oauthProviders = [];
|
|
foreach (config('auth.oauth') as $name => $data) {
|
|
config()->set("services.$name", array_merge($data['service'], ['redirect' => "/auth/oauth/callback/$name"]));
|
|
|
|
if (isset($data['provider'])) {
|
|
$oauthProviders[$name] = $data['provider'];
|
|
}
|
|
}
|
|
|
|
Event::listen(function (SocialiteWasCalled $event) use ($oauthProviders) {
|
|
foreach ($oauthProviders as $name => $provider) {
|
|
$event->extendSocialite($name, $provider);
|
|
}
|
|
});
|
|
|
|
FilamentColor::register([
|
|
'danger' => Color::Red,
|
|
'gray' => Color::Zinc,
|
|
'info' => Color::Sky,
|
|
'primary' => Color::Blue,
|
|
'success' => Color::Green,
|
|
'warning' => Color::Amber,
|
|
]);
|
|
|
|
FilamentView::registerRenderHook(
|
|
PanelsRenderHook::CONTENT_START,
|
|
fn () => view('filament.server-conflict-banner'),
|
|
scopes: Console::class,
|
|
);
|
|
|
|
// Don't run any health checks during tests
|
|
if (!$app->runningUnitTests()) {
|
|
Health::checks([
|
|
DebugModeCheck::new()->if($app->isProduction()),
|
|
EnvironmentCheck::new(),
|
|
CacheCheck::new(),
|
|
DatabaseCheck::new(),
|
|
ScheduleCheck::new(),
|
|
UsedDiskSpaceCheck::new(),
|
|
PanelVersionCheck::new(),
|
|
NodeVersionsCheck::new(),
|
|
]);
|
|
}
|
|
|
|
Gate::before(function (User $user, $ability) {
|
|
return $user->isRootAdmin() ? true : null;
|
|
});
|
|
|
|
AboutCommand::add('Pelican', [
|
|
'Panel Version' => $versionService->currentPanelVersion(),
|
|
'Latest Version' => $versionService->latestPanelVersion(),
|
|
'Up-to-Date' => $versionService->isLatestPanel() ? '<fg=green;options=bold>Yes</>' : '<fg=red;options=bold>No</>',
|
|
]);
|
|
|
|
AboutCommand::add('Drivers', 'Backups', config('backups.default'));
|
|
|
|
AboutCommand::add('Environment', 'Installation Directory', base_path());
|
|
}
|
|
|
|
/**
|
|
* Register application service providers.
|
|
*/
|
|
public function register(): void
|
|
{
|
|
Scramble::extendOpenApi(fn (OpenApi $openApi) => $openApi->secure(SecurityScheme::http('bearer')));
|
|
Scramble::ignoreDefaultRoutes();
|
|
}
|
|
}
|