mirror of
https://github.com/pelican-dev/panel.git
synced 2025-05-19 22:14:45 +02:00
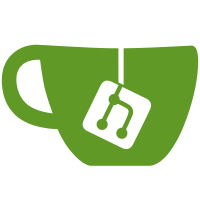
* add spatie health * change slug for health page * add check for panel version * only check for debug mode if env isn't local * add check for node versions * improve short summary * fix outdated check * run pint * fix health checks during tests * add count to ok message * fix typo * temp fix for phpstan job * fix pint... * improve "outdated" count Co-authored-by: MartinOscar <40749467+RMartinOscar@users.noreply.github.com> * run pint * skip node versions check if no nodes are created * auto run health checks if they didn't run before * small refactor * update navigation Co-authored-by: Charles <sir3lit@gmail.com> * fix errors if tests didn't run yet * fix disk usage check * remove plugin and use own page * use health status indicator from spatie * fix after merge * update icon * update color classes * fix after merge * add back imports oops... * wrong import oops²... * update spatie/laravel-health to latest * move Health page to correct namespace * update NodeVersionsCheck * use style instead of tailwind classes workaround until we have vite * cleanup custom checks --------- Co-authored-by: MartinOscar <40749467+RMartinOscar@users.noreply.github.com> Co-authored-by: Charles <sir3lit@gmail.com>
121 lines
3.1 KiB
PHP
121 lines
3.1 KiB
PHP
<?php
|
|
|
|
namespace App\Filament\Admin\Pages;
|
|
|
|
use Carbon\Carbon;
|
|
use Filament\Actions\Action;
|
|
use Filament\Notifications\Notification;
|
|
use Filament\Pages\Page;
|
|
use Illuminate\Support\Facades\Artisan;
|
|
use Spatie\Health\Commands\RunHealthChecksCommand;
|
|
use Spatie\Health\ResultStores\ResultStore;
|
|
|
|
class Health extends Page
|
|
{
|
|
protected static ?string $navigationIcon = 'tabler-heart';
|
|
|
|
protected static ?string $navigationGroup = 'Advanced';
|
|
|
|
protected static string $view = 'filament.pages.health';
|
|
|
|
// @phpstan-ignore-next-line
|
|
protected $listeners = [
|
|
'refresh-component' => '$refresh',
|
|
];
|
|
|
|
protected function getActions(): array
|
|
{
|
|
return [
|
|
Action::make('refresh')
|
|
->button()
|
|
->action('refresh'),
|
|
];
|
|
}
|
|
|
|
protected function getViewData(): array
|
|
{
|
|
// @phpstan-ignore-next-line
|
|
$checkResults = app(ResultStore::class)->latestResults();
|
|
|
|
if ($checkResults === null) {
|
|
Artisan::call(RunHealthChecksCommand::class);
|
|
|
|
$this->dispatch('refresh-component');
|
|
}
|
|
|
|
return [
|
|
'lastRanAt' => new Carbon($checkResults?->finishedAt),
|
|
'checkResults' => $checkResults,
|
|
];
|
|
}
|
|
|
|
public function refresh(): void
|
|
{
|
|
Artisan::call(RunHealthChecksCommand::class);
|
|
|
|
$this->dispatch('refresh-component');
|
|
|
|
Notification::make()
|
|
->title('Health check results refreshed')
|
|
->success()
|
|
->send();
|
|
}
|
|
|
|
public static function getNavigationBadge(): ?string
|
|
{
|
|
// @phpstan-ignore-next-line
|
|
$results = app(ResultStore::class)->latestResults();
|
|
|
|
if ($results === null) {
|
|
return null;
|
|
}
|
|
|
|
$results = json_decode($results->toJson(), true);
|
|
|
|
$failed = array_reduce($results['checkResults'], function ($numFailed, $result) {
|
|
return $numFailed + ($result['status'] === 'failed' ? 1 : 0);
|
|
}, 0);
|
|
|
|
return $failed === 0 ? null : (string) $failed;
|
|
}
|
|
|
|
public static function getNavigationBadgeColor(): string
|
|
{
|
|
return self::getNavigationBadge() > null ? 'danger' : '';
|
|
}
|
|
|
|
public static function getNavigationBadgeTooltip(): ?string
|
|
{
|
|
// @phpstan-ignore-next-line
|
|
$results = app(ResultStore::class)->latestResults();
|
|
|
|
if ($results === null) {
|
|
return null;
|
|
}
|
|
|
|
$results = json_decode($results->toJson(), true);
|
|
|
|
$failedNames = array_reduce($results['checkResults'], function ($carry, $result) {
|
|
if ($result['status'] === 'failed') {
|
|
$carry[] = $result['name'];
|
|
}
|
|
|
|
return $carry;
|
|
}, []);
|
|
|
|
return 'Failed: ' . implode(', ', $failedNames);
|
|
}
|
|
|
|
public static function getNavigationIcon(): string
|
|
{
|
|
// @phpstan-ignore-next-line
|
|
$results = app(ResultStore::class)->latestResults();
|
|
|
|
if ($results === null) {
|
|
return 'tabler-heart-question';
|
|
}
|
|
|
|
return $results->containsFailingCheck() ? 'tabler-heart-exclamation' : 'tabler-heart-check';
|
|
}
|
|
}
|