mirror of
https://github.com/pelican-dev/panel.git
synced 2025-07-04 09:31:07 +02:00
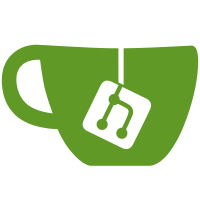
* add spatie health * change slug for health page * add check for panel version * only check for debug mode if env isn't local * add check for node versions * improve short summary * fix outdated check * run pint * fix health checks during tests * add count to ok message * fix typo * temp fix for phpstan job * fix pint... * improve "outdated" count Co-authored-by: MartinOscar <40749467+RMartinOscar@users.noreply.github.com> * run pint * skip node versions check if no nodes are created * auto run health checks if they didn't run before * small refactor * update navigation Co-authored-by: Charles <sir3lit@gmail.com> * fix errors if tests didn't run yet * fix disk usage check * remove plugin and use own page * use health status indicator from spatie * fix after merge * update icon * update color classes * fix after merge * add back imports oops... * wrong import oops²... * update spatie/laravel-health to latest * move Health page to correct namespace * update NodeVersionsCheck * use style instead of tailwind classes workaround until we have vite * cleanup custom checks --------- Co-authored-by: MartinOscar <40749467+RMartinOscar@users.noreply.github.com> Co-authored-by: Charles <sir3lit@gmail.com>
44 lines
1.3 KiB
PHP
44 lines
1.3 KiB
PHP
<?php
|
|
|
|
namespace App\Checks;
|
|
|
|
use App\Models\Node;
|
|
use App\Services\Helpers\SoftwareVersionService;
|
|
use Spatie\Health\Checks\Check;
|
|
use Spatie\Health\Checks\Result;
|
|
use Spatie\Health\Enums\Status;
|
|
|
|
class NodeVersionsCheck extends Check
|
|
{
|
|
public function __construct(private SoftwareVersionService $versionService) {}
|
|
|
|
public function run(): Result
|
|
{
|
|
$all = Node::query()->count();
|
|
|
|
if ($all === 0) {
|
|
$result = Result::make()->notificationMessage('No Nodes created')->shortSummary('No Nodes');
|
|
$result->status = Status::skipped();
|
|
|
|
return $result;
|
|
}
|
|
|
|
$latestVersion = $this->versionService->latestWingsVersion();
|
|
|
|
$outdated = Node::query()->get()
|
|
->filter(fn (Node $node) => !isset($node->systemInformation()['exception']) && $node->systemInformation()['version'] !== $latestVersion)
|
|
->count();
|
|
|
|
$result = Result::make()
|
|
->meta([
|
|
'all' => $all,
|
|
'outdated' => $outdated,
|
|
])
|
|
->shortSummary($outdated === 0 ? 'All up-to-date' : "{$outdated}/{$all} outdated");
|
|
|
|
return $outdated === 0
|
|
? $result->ok('All Nodes are up-to-date.')
|
|
: $result->failed(':outdated/:all Nodes are outdated.');
|
|
}
|
|
}
|